Here's an alternative implementation. This one puts the commas into the input fields, puts .00 at the end of the input if the user omits it, and converts anything that's not a number to 0.00:
$(document).ready(function() {
function applyCurrencyFormat(field) {
var currentValue = parseNumber($(field).val());
var formattedValue = currentValue.toFixed(2).replace(/(\d)(?=(\d{3})+\.)/g, '$1,');
$(field).val(formattedValue);
}
function removeCurrencyFormat(field) {
var currentValue = $(field).val();
var formattedValue = parseNumber(currentValue.replace(/,/g,'')).toFixed(2);
$(field).val(formattedValue);
}
$('.sum input').removeAttr('pattern');
$('.sum input').on('blur', function() {
$('.sum input').each(function() {
removeCurrencyFormat(this);
});
sumtotal();
$('.sum input').each(function() {
applyCurrencyFormat(this);
});
});
function sumtotal() {
var s = 0;
$('.sum input').each(function () {
s += parseNumber($(this).val());
});
$('.total input').val(s.toFixed(2).replace(/(\d)(?=(\d{3})+\.)/g, '$1,'));
}
function parseNumber(n) {
var f = parseFloat(n); //Convert to float number.
return isNaN(f) ? 0 : f; //treat invalid input as 0;
}
});
Below is the class setup:
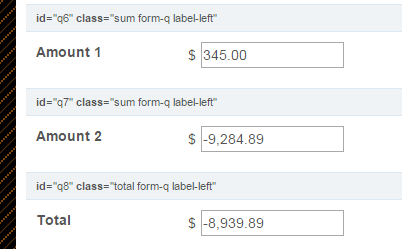