Here is an example using your sample form:
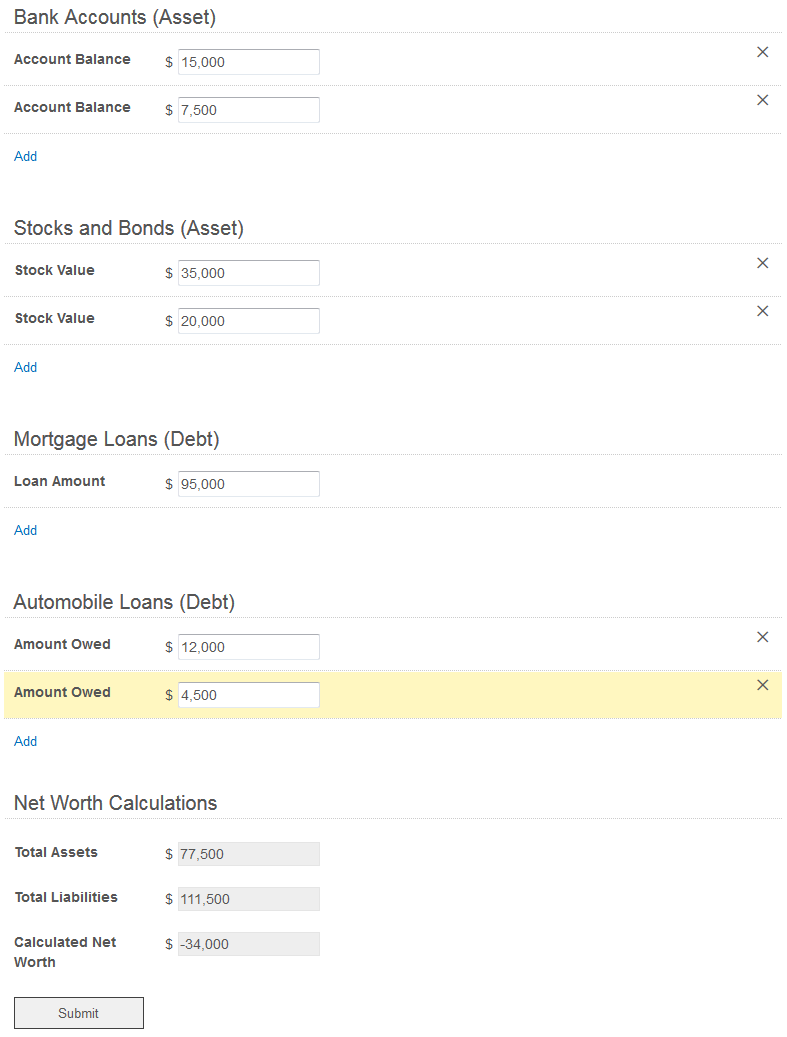
And here is the Javascript I'm using
$(document).ready(function () {
//set net worth calculation fields to be read-only
$('.totalassets input').attr('readonly','True');
$('.totaldebt input').attr('readonly','True');
$('.networth input').attr('readonly','True');
//initialize currency input fields
setfields();
//handle adding more items in collections
$('.cf-collection-append').on('click', setfields);
//handle removing items from collections
$(document).on('click', sumtotal);
//changes input fields to allow for commas and replaces the input with the comma formatted value
function setfields() {
$('.commas input').attr("pattern", '^(\\d+|\\d{1,3}(,\\d{3})*)(\\.\\d{2})?$');
$('.commas input').on("change", function () {
$(this).val($(this).val().replace(/(\d)(?=(\d\d\d)+(?!\d))/g, "$1,"));
});
$('.sumasset, .sumdebt').on('blur', 'input', sumtotal);
}
//calculates total assets and liabilities
function sumtotal() {
var sa = 0;
var sd = 0;
$('.sumasset input').each(function () {
sa += parseNumber($(this).val().replace(/,/g,''));
});
$('.totalassets input').val(sa.toString().replace(/(\d)(?=(\d\d\d)+(?!\d))/g, "$1,"));
$('.totalassets input').trigger('change');
$('.sumdebt input').each(function () {
sd += parseNumber($(this).val().replace(/,/g,''));
});
$('.totaldebt input').val(sd.toString().replace(/(\d)(?=(\d\d\d)+(?!\d))/g, "$1,"));
$('.totaldebt input').trigger('change');
}
//calculates total net worth
$('.totalassets input, .totaldebt input').on('change', function() {
var a = parseNumber($('.totalassets input').val().replace(/,/g,''));
var d = parseNumber($('.totaldebt input').val().replace(/,/g,''));
var n = a - d;
$('.networth input').val(n.toString().replace(/(\d)(?=(\d\d\d)+(?!\d))/g, "$1,"));
});
function parseNumber(n) {
var f = parseFloat(n); //Convert to float number.
return isNaN(f) ? 0 : f; //treat invalid input as 0;
}
});
The account balance field uses the CSS class, commas sumasset
The stock value field uses the CSS class, commas sumasset
The loan value field uses the CSS class, commas sumdebt
The amount owed field uses the CSS class, commas sumdebt
The total assets field uses the CSS class, commas totalassets
The total liabilities field uses the CSS class, commas totaldebt
The total net worth field uses the CSS class, commas networth