Here's another option @████████
This is a tweak of the original script. But I moved the creation of the backout dates array to before we set the start and end dates of the date field. Then when we are setting the start date of the field, we're going to loop through 3 times. Each iteration of the loop, we're going to add 1 day. Then we'll check if that new day is a weekend or in the blackout dates array, if it is, we reduce the increment count by 1 so that it runs the loop an extra time.
This is successfully working for me on Friday 6/16/2023 - with Saturday, Sunday, and the Monday Holiday (Juneteenth National Independence Day) being excluded, and saying the 3rd business day is Thursday the 22nd.
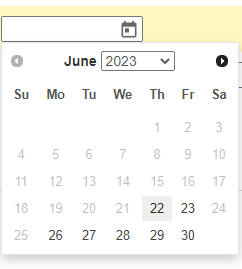
//create the array of blackout dates
var blackoutDatesList = new Array();
//this function is run when the form is loaded
$(document).ready(function(){
//loop through each date in the blackout date list, and add it to an array
blackoutDatesList.length = 0;
$('.blackoutDates option').each(function() {
blackoutDatesList.push(formatDate(new Date($(this).val())));
});
//set the date range of the Date field between 3 business days and 30 days in the future
var today = new Date();
var startDate = new Date();
for(i = 1; i <= 3; i++) {
startDate.setDate(startDate.getDate() + 1);
var t = startDate.getDay();
var string = jQuery.datepicker.formatDate('yy-mm-dd', startDate);
if (t==6 || t==0 || blackoutDatesList.indexOf(string) != -1) {
i--;
}
}
var endDate = new Date();
endDate.setDate(today.getDate() + 30);
var minDate = $.datepicker.formatDate('yy-mm-dd', new Date(startDate));
var maxDate = $.datepicker.formatDate('yy-mm-dd', new Date(endDate));
$('.myLimitedDate input').attr('min',minDate);
$('.myLimitedDate input').attr('max',maxDate);
//the following four function work together to disable any dates on the picker
//that are weekends or are on the blackoutDatesList array
//they also add a validation (parsley) setting for invalid date entries
function isValidDate(n) {
var t = n.getDay();
var d = n.getDate();
var string = jQuery.datepicker.formatDate('yy-mm-dd', n);
return (t!=6 && t!=0 && blackoutDatesList.indexOf(string) == -1);
}
function checkDate(n) {
return[isValidDate(n),""];
}
function checkField(input, inst) {
if ($(input).closest('li').hasClass('myLimitedDate')) {
$(input).datepicker("option", {beforeShowDay: checkDate});
}
}
$.datepicker.setDefaults( {beforeShow: checkField} );
window.Parsley.addValidator('limiteddate', {
validateString: function(value) {
//IMPORTANT! this date format needs to match the format you set for the field on the layout page
return isValidDate(moment(value, "YYYY-MM-DD").toDate());
},
messages: {
en: 'Not a valid date.'
}
});
$('.myLimitedDate input').attr('data-parsley-limiteddate','');
});
//this function is run when the lookups are completed.
$(document).on("onloadlookupfinished", function () {
//loop through each date in the blackout date list, and add it to an array
blackoutDatesList.length = 0;
$('.blackoutDates option').each(function() {
blackoutDatesList.push(formatDate(new Date($(this).val())));
});
});
//function to return any date formatted as yyyy-mm-dd
function formatDate(date) {
var d = new Date(date),
month = '' + (d.getMonth() + 1),
day = '' + (d.getDate() +1),
year = d.getFullYear();
if (month.length < 2)
month = '0' + month;
if (day.length < 2)
day = '0' + day;
return [year, month, day].join('-');
}