Thanks for your responses.
Here is the code I'm using
namespace WorkflowActivity.Scripting.CreaDocumento
{
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Data.SqlClient;
using System.Linq;
using System.Text;
using System.IO;
using System.Reflection;
using Microsoft.Office.Core;
using System.Windows.Forms;
using Word = Microsoft.Office.Interop.Word;
/// <summary>
/// Provides one or more methods that can be run when the workflow scripting activity is performed.
/// </summary>
public class Script1 : ScriptClass90
{
/// <summary>
/// This method is run when the activity is performed.
/// </summary>
protected override void Execute()
{
// Write your code here. The BoundEntryInfo property will access the entry, RASession will get the Repository Access session
Word.Application wordApp;
Word.Document aDoc;
object missing = Missing.Value;
object filename;
string nombre = "Documento de Prueba";
string docPath = @"C:\Firma Digital (no borrar)\Documentos\Machote\Machote prueba.docx";
string ruta = @"C:\Firma Digital (no borrar)\Documentos\En Proceso\"+nombre+".docx";
string ruta2 = @"C:\Firma Digital (no borrar)\Documentos\Terminada\"+nombre+".docx";
File.Copy(docPath, Path.Combine(Application.StartupPath, ruta),true);
wordApp = new Word.Application();
filename = Path.Combine(Application.StartupPath,ruta);
if(File.Exists((string)filename)){
object readOnly = false;
object isVisible = false;
wordApp.Visible = false;
aDoc = wordApp.Documents.Open(ref filename, ref missing, ref readOnly,
ref missing, ref missing, ref missing,
ref missing, ref missing, ref missing,
ref missing, ref missing, ref isVisible,
ref missing, ref missing, ref missing,
ref missing);
aDoc.Activate();
string f = "";
string r = "";
for(int i = 1; i <= 2; i++){
f = "";
r = "";
if(i == 1){
f = "<nombre>";
r = "Ricardo";
}
if(i == 2){
f = "<cedula>";
r = "207140375";
}
object matchCase = true;
object matchWholeWord = true;
object matchWildCards = false;
object matchSoundsLike = false;
object matchAllWordForms = false;
object forward = true;
object format = false;
object matchKashida = false;
object matchDiacritics = false;
object matchAlefHamza = false;
object matchControl = false;
object read_only = false;
object visible = true;
object replace = 2;
object wrap = 1;
object findText = f; //<------------Variable f
object replaceText = r; //<------------Variable r
wordApp.Selection.Find.Execute(ref findText, ref matchCase, ref matchWholeWord,
ref matchWildCards, ref matchSoundsLike, ref matchAllWordForms,
ref forward, ref wrap, ref format, ref replaceText, ref replace,
ref matchKashida, ref matchDiacritics, ref matchAlefHamza, ref matchControl);
}
aDoc.Save();
object saveChanges = Word.WdSaveOptions.wdSaveChanges;
wordApp.Quit(ref saveChanges, ref missing, ref missing);
}
}
}
}
And here are the references
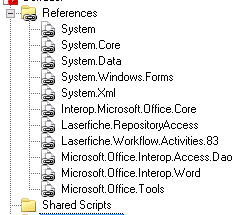
The code is not really well made, but I feel Script Editor isn't as flexible as other IDEs.
Carl, the dll files are located in a "C" path, so when I added the references I pick them from there, how can I know if WF can access to that path? By IIS?
Regards!