Nate, here's something that should work.
Place a hidden single line field at the top of your form. Let's assume it is called "Hidden Reason" and has an id of #q1.
Then insert this snippet into the JavaScript/CSS section (you can ignore the first and last line if you already have them):
$(document).ready(function() {
var reasons = $('#q1 input').val().split(",");
$.each(reasons, function(index, value) {
$("input[value='" + value + "']").attr('checked', true);
});
});
Then structure your URL to look like this:
myServer/Forms/form/new/24?Hidden_Reason=New_Hire,Promotion,Dept._Change
The bolded stuff is the values you want, and they are separated by commas. When the form loads, the hidden field is populated, then the code snippet above iterates through each value, finds its checkbox and checks it:
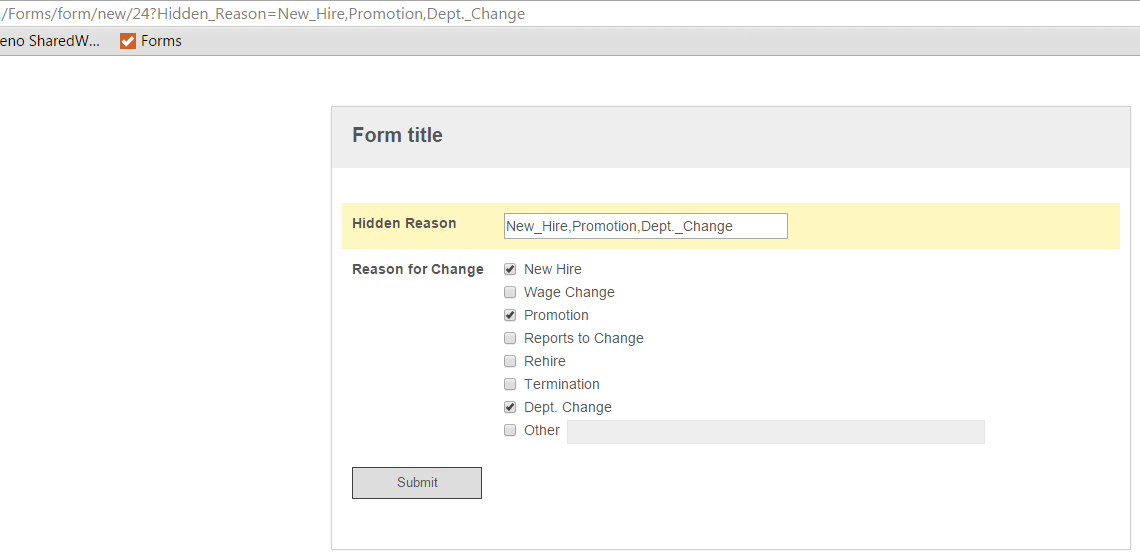
If you also need to populate the "Other" option using the URL, you can use the expanded version of the snippet below:
$(document).ready(function() {
var reasons = $('#q1 input').val().split(",");
var otherInput = $("#Field2_other_value");
$.each(reasons, function(index, value) {
if (value.lastIndexOf("Other;", 0) === 0) {
var otherReason = value.split("Other;")[1];
$("#Field2_other").prop("checked", true);
otherInput.attr({
disabled: false,
readonly: false
}).val(otherReason);
} else {
$("input[value='" + value + "']").attr('checked', true);
}
});
});
The only differences here is that in your URL, the "Other" reason has to be separated using a semicolon instead of a comma, so it should look like this:
myServer/Forms/form/new/24?Hidden_Reason=New_Hire,Promotion,Dept._Change,Other;myreason
And inside the code snippet, you need to target the Other checkbox and its input field using their ids. In my case they're #Field2_other and #Field2_other_value.
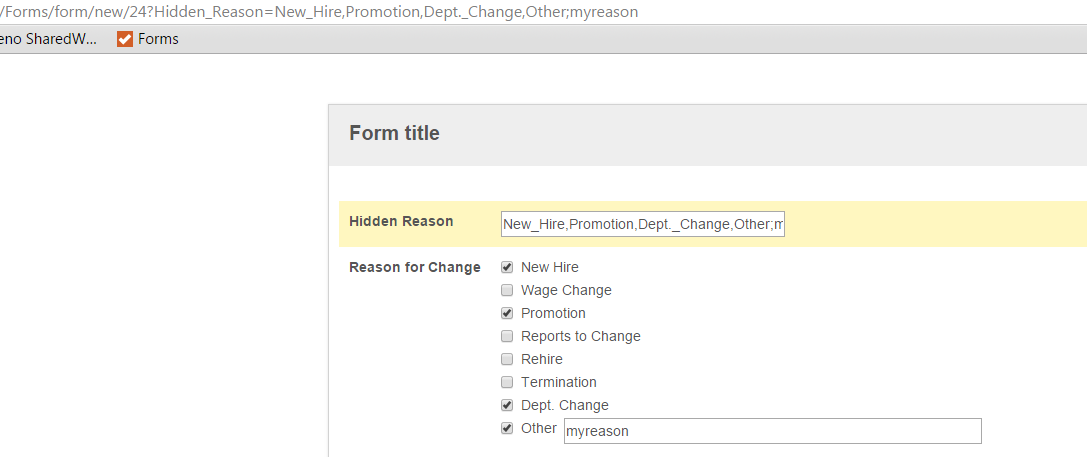
If you need to include spaces in your "Other" reason, use %20. HTML URL Encoding Reference