SELECTED ANSWER
replied on November 5, 2015
One option is to first check the length and then check the complexity requirements and make sure that at least three out of four are used. Try the JavaScript below. The password field on the form will need the CSS class name, pwd.
$(document).ready(function () {
$('.pwd input').on('blur', function() {
var minMaxLength = /^[\s\S]{8,32}$/;
var upper = /[A-Z]/;
var lower = /[a-z]/;
var number = /[0-9]/;
var special = /[^A-Za-z0-9]/;
var count = 0;
$('p.complexityWarning').remove();
$('p.lengthWarning').remove();
if (minMaxLength.test($(this).val())) {
$('p.lengthWarning').remove();
if (upper.test($(this).val())) count++;
if (lower.test($(this).val())) count++;
if (number.test($(this).val())) count++;
if (special.test($(this).val())) count++;
if (count < 3) {
$('<p class="complexityWarning">The password does not meet the complexity requirements.</p>').insertAfter('.pwd');
$('.Submit').attr('disabled', true);
} else {
$('p.complexityWarning').remove();
$('.Submit').removeAttr('disabled');
}
} else {
if (upper.test($(this).val())) count++;
if (lower.test($(this).val())) count++;
if (number.test($(this).val())) count++;
if (special.test($(this).val())) count++;
if (count < 3) {
$('<p class="complexityWarning">The password does not meet the complexity requirements.</p>').insertAfter('.pwd');
} else {
$('p.complexityWarning').remove();
}
$('<p class="lengthWarning">The password is not between 8 and 32 characters.</p>').insertAfter('.pwd');
$('.Submit').attr('disabled', true);
}
});
});
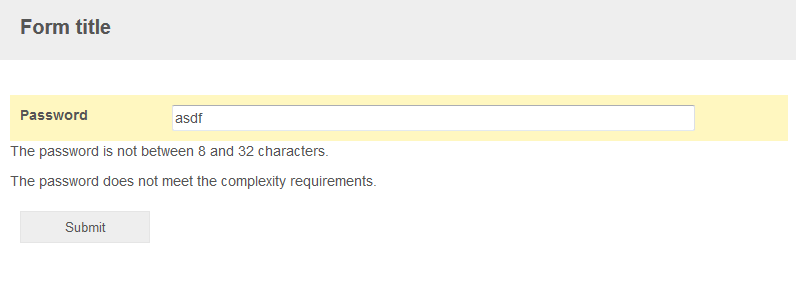
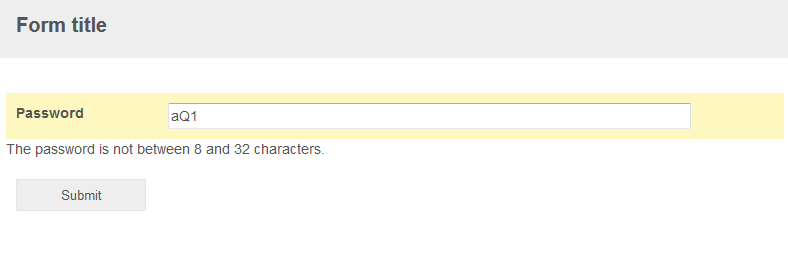
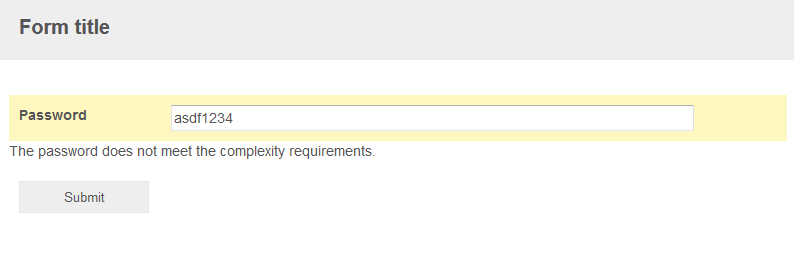
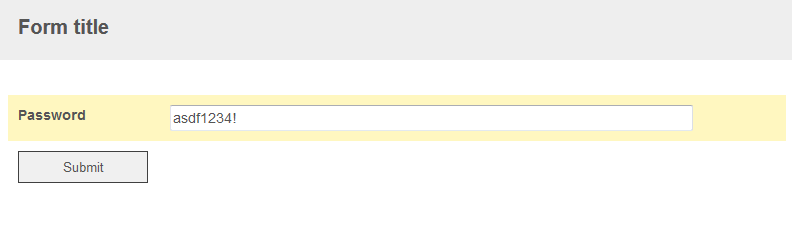