I know this is an old feed but wanted to post this on here in case this helps anyone else.
I am recently new to Laserfiche and inherited a repository that needed to have a lot of clean up. I wanted a way to find all documents that said they had a document assigned to it but like the original post stated "Couldn't find text or image for page". I created a work flow to 1st query the Laserfiche repository. Then foreach of those entries ran I ran a query to get the Windows file location of the file. I then used a C# script to check if file existed if it did it updates a token for a report, and if it didn't exist it did the same updated a different token that would create a different report. This would allow me to view the documents in Laserfiche Repository that didn't have an actual windows file associated to it.
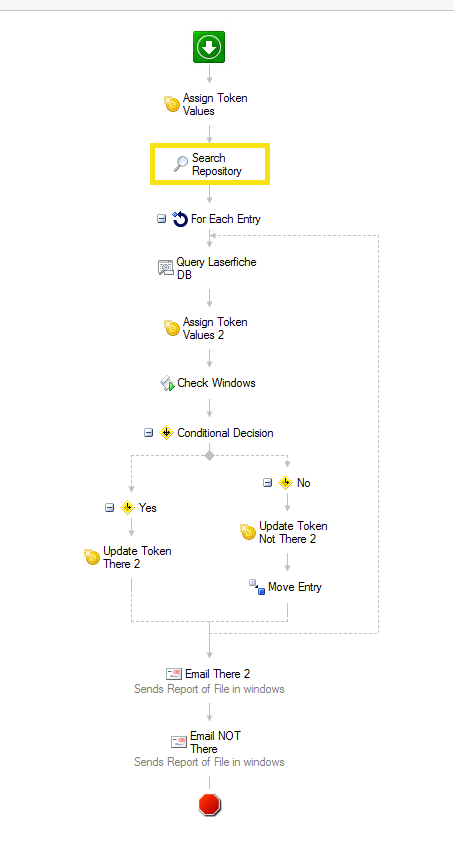
This is the query I used to get where the file was from the LF database.
SELECT dbo.toc.name AS DocumentName, dbo.doc.pagenum + 1 AS PageNum, dbo.vol.fixpath + '\' +
SUBSTRING(CONVERT(VARCHAR(8),CONVERT(VARBINARY(4), dbo.doc.storeid),2),1,2) + '\' +
SUBSTRING(CONVERT(VARCHAR(8),CONVERT(VARBINARY(4), dbo.doc.storeid),2),3,2) + '\' +
SUBSTRING(CONVERT(VARCHAR(8),CONVERT(VARBINARY(4), dbo.doc.storeid),2),5,2) + '\' +
CONVERT(VARCHAR(8),CONVERT(VARBINARY(4), dbo.doc.storeid),2) + '.TIF' AS FullPathAndFilename
FROM dbo.doc
LEFT JOIN dbo.toc ON dbo.doc.tocid = dbo.toc.tocid
LEFT JOIN dbo.vol ON dbo.toc.vol_id = dbo.vol.vol_id
WHERE dbo.doc.tocid = ?
ORDER BY dbo.doc.pagenum
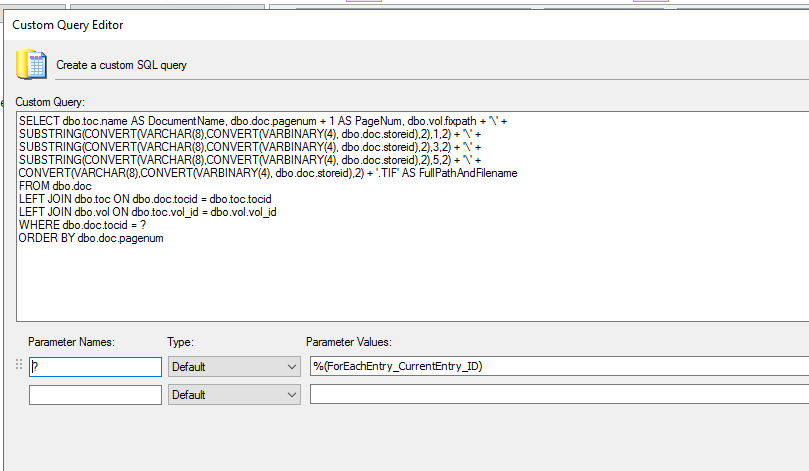
This is the script I used to check if the file existed.
namespace WorkflowActivity.Scripting.CheckWindows
{
using System;
using System.IO;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Data.SqlClient;
using System.Text;
using Laserfiche.RepositoryAccess;
using Laserfiche.DocumentServices;
/// <summary>
/// Provides one or more methods that can be run when the workflow scripting activity is performed.
/// </summary>
public class Script1 : RAScriptClass104
{
/// <summary>
/// This method is run when the activity is performed.
/// </summary>
protected override void Execute()
{
// Write your code here. The BoundEntryInfo property will access the entry, RASession will get the Repository Access session
//try
//{
// Get Path to Image
String ImageLocation = (String)GetTokenValue("WindowsPath");
// Update Token if File Exists
//System.IO.DirectoryInfo di = new System.IO.DirectoryInfo(WindowsPath);
if (File.Exists(@ImageLocation))
{
SetActivityTokenValue("WindowPathTrue",true);
Console.WriteLine("Yes file is there");
}
else
{
SetActivityTokenValue("WindowPathTrue",false);
Console.WriteLine("No file is there");
}
//}
}}}
Hope this might help someone else.