For those interested in the project code, here is what I did. First this is my form design.
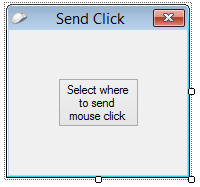
Here is the VB code (Target Framework = .Net 4.0):
Imports System.Text
Public Class Form1
' Delegates for enum callbacks
Private Delegate Function EnumChildProc(ByVal hWnd As IntPtr, ByRef data As WindowData) As Int32
Private Delegate Function EnumWindowsProc(ByVal hWnd As IntPtr, ByRef data As WindowData) As Boolean
' Win32API Calls
Private Declare Function EnumChildWindows Lib "USER32" (ByVal hwndParent As IntPtr, ByVal lpEnumFunc As [Delegate], ByRef data As WindowData) As Integer
Private Declare Function EnumWindows Lib "USER32" (ByVal callback As EnumWindowsProc, ByRef data As WindowData) As Boolean
Private Declare Function GetCursorPos Lib "USER32" (ByRef lpPoint As POINTAPI) As Integer
Private Declare Function WindowFromPointXY Lib "USER32" Alias "WindowFromPoint" (ByVal xPoint As Integer, ByVal yPoint As Integer) As IntPtr
Private Declare Function GetAncestor Lib "USER32" (ByVal hwnd As IntPtr, ByVal gaFlags As Integer) As IntPtr
Private Declare Function GetWindowText Lib "USER32" Alias "GetWindowTextA" (ByVal hwnd As IntPtr, ByVal lpString As StringBuilder, ByVal cch As Integer) As Integer
Private Declare Function GetClassName Lib "USER32" Alias "GetClassNameA" (ByVal hwnd As IntPtr, ByVal lpClassName As StringBuilder, ByVal nMaxCount As Integer) As Integer
Private Declare Function GetWindowLong Lib "USER32" Alias "GetWindowLongA" (ByVal hwnd As IntPtr, ByVal nIndex As Integer) As Integer
Private Declare Function SendMessage Lib "USER32" Alias "SendMessageW" (ByVal hwnd As IntPtr, ByVal wMsg As Integer, ByVal wParam As Integer, ByVal lParam As Integer) As Integer
Private Declare Function SendMessage Lib "USER32" Alias "SendMessageW" (ByVal hwnd As IntPtr, ByVal wMsg As Integer, ByVal wParam As Integer, ByVal lParam As StringBuilder) As Integer
Private Declare Function SendMessage Lib "USER32" Alias "SendMessageW" (ByVal hwnd As IntPtr, ByVal wMsg As Integer, ByVal wParam As Integer, ByVal lParam As String) As Integer
Private Declare Function SendMessage Lib "USER32" Alias "SendMessageW" (ByVal hwnd As IntPtr, ByVal wMsg As Integer, ByVal wParam As Integer, ByVal lParam As IntPtr) As Integer
Private Declare Function PostMessage Lib "USER32" Alias "PostMessageA" (ByVal hWnd As System.IntPtr, ByVal Msg As UInteger, ByVal wParam As IntPtr, ByVal lParam As IntPtr) As Boolean
Private Declare Function GetWindowRect Lib "USER32" (ByVal hWnd As IntPtr, ByRef lpRect As RECT) As Boolean
' Constants
Private Const GA_ROOT As Integer = 2
Private Const GWL_STYLE As Integer = (-16)
Private Const WM_SETTEXT As Integer = &HC
Private Const WM_KEYDOWN As Integer = &H100
Private Const WM_KEYUP As Integer = &H101
Public Const WM_LBUTTONDOWN As Integer = 513
Public Const WM_LBUTTONUP As Integer = 514
' This will hold vars for finding the target...
Private Class WindowData
' Window Caption
Public sWindowText As String
' Top Window Caption
Public sTopWindowText As String
' Window Class
Public sWindowClass As String
' Top Window Class
Public sTopWindowClass As String
' Handle of Window
Public hWnd As IntPtr
' Handle of Top Window
Public TophWnd As IntPtr
' Window Style
Public iWindowStyle As Integer
' Window Index
Public iWindowIndex As Integer
' Current index
Public iCurIndex As Integer
End Class
Private Structure RECT
Dim Left As Integer ' // x position of upper-left corner
Dim Top As Integer ' // y position of upper-left corner
Dim Right As Integer ' // x position of lower-right corner
Dim Bottom As Integer ' // y position of lower-right corner
End Structure
Private Structure POINTAPI
Dim x As Integer
Dim y As Integer
End Structure
' Function to get information about a subwindow
Private Function GetData() As WindowData
Dim wd As New WindowData
Dim MaxLen As Integer = 255
Dim pt32 As POINTAPI
Dim sParentText As New StringBuilder(MaxLen)
Dim sWindowText As New StringBuilder(MaxLen)
Dim sClassName As New StringBuilder(MaxLen)
Dim hWndOver As IntPtr
Dim lWindowStyle As Integer
Dim iResult As Integer
Try
' Get cursor position
GetCursorPos(pt32)
' Get window cursor is over
hWndOver = WindowFromPointXY(pt32.x, pt32.y)
' Only Process if window handle is not 0
If hWndOver <> IntPtr.Zero Then
wd.hWnd = hWndOver
Try
' Get handle for main window
Dim iTop As IntPtr = GetAncestor(hWndOver, GA_ROOT)
' Process main window only if handle is not 0
If iTop <> IntPtr.Zero Then
wd.TophWnd = iTop
' Get Top Window text
iResult = GetWindowText(iTop, sParentText, MaxLen)
wd.sTopWindowText = sParentText.ToString
' Get Top Window Class
iResult = GetClassName(iTop, sParentText, MaxLen)
wd.sTopWindowClass = sParentText.ToString
End If
' Get Window text
iResult = GetWindowText(hWndOver, sWindowText, MaxLen)
wd.sWindowText = sWindowText.ToString
Catch ex As Exception
End Try
Try
' Get Window Class
iResult = GetClassName(hWndOver, sClassName, MaxLen)
wd.sWindowClass = sClassName.ToString
Catch ex As Exception
End Try
Try
' Get Window Style
lWindowStyle = GetWindowLong(hWndOver, GWL_STYLE)
wd.iWindowStyle = lWindowStyle.ToString
Catch ex As Exception
End Try
Try
wd.iWindowIndex = GetIndex(wd.TophWnd, wd.hWnd, wd.sWindowClass)
Catch ex As Exception
End Try
End If
Catch ex As Exception
MsgBox(ex.Message)
End Try
Return wd
End Function
#Region "Enums"
Private Function GetIndex(ByVal ParentHandle As IntPtr, ByVal TargetHandle As IntPtr, ByVal ClassName As String) As Integer
Dim cd As New WindowData()
cd.iWindowIndex = 0
'Only process if provided handle is not Zero
If ParentHandle <> IntPtr.Zero And TargetHandle <> IntPtr.Zero Then
With cd
'Set target class
.sWindowClass = ClassName
'Set target index
.iWindowIndex = 0
'Zero current index
.iCurIndex = 0
'Zero Handle
.hWnd = TargetHandle
End With
' Setup new enum
Dim myEnumChildProc As New EnumChildProc(AddressOf EnumChildFindIndex)
' Enum childwindows
EnumChildWindows(ParentHandle, myEnumChildProc, cd)
End If
Return cd.iWindowIndex
End Function
'Call back for EnumChildWindows
Private Function EnumChildFindIndex(ByVal hWnd As IntPtr, ByRef data As WindowData) As Int32
Dim ClassName As New StringBuilder(1024)
' Get the Child class name
GetClassName(hWnd, ClassName, ClassName.Capacity)
'Compair if its the class name we are looking for
'Use .Contains to allow partial Class match
'If ClassName.ToString().Contains(data.sWinClass) Then
'Use = to require perfect Class match
If ClassName.ToString() = data.sWindowClass Then
' Class found - Increase Current Index by 1
data.iCurIndex += 1
' Check if class is at correct index
If data.hWnd = hWnd Then
data.iWindowIndex = data.iCurIndex
'Stop Enum
Return 0
End If
End If
'Continue Enum
Return 1
End Function
Private Function GetChild(ByVal MainClass As String, ByVal MainCaption As String, ByVal ChildClass As String, ByVal ChildIndex As Integer) As IntPtr
'Get Handle Of Main Window
Dim iMain As IntPtr = FindMainWindow(MainClass, MainCaption)
'Only continue if Main window found
If iMain <> IntPtr.Zero Then
'Set our ChildData
Dim cd As New WindowData()
With cd
'Set target class
.sWindowClass = ChildClass
'Set target index
.iWindowIndex = ChildIndex
'Zero current index
.iCurIndex = 0
'Zero Handle
.hWnd = IntPtr.Zero
End With
' Setup new enum
Dim myEnumChildProc As New EnumChildProc(AddressOf EnumChild)
' Enum childwindows
EnumChildWindows(iMain, myEnumChildProc, cd)
'Return window handle
Return cd.hWnd
End If
'return Zero
Return IntPtr.Zero
End Function
Private Function GetChild(ByVal ParentHandle As IntPtr, ByVal ChildClass As String, ByVal ChildIndex As Integer) As IntPtr
'Only process if provided handle is not Zero
If ParentHandle <> IntPtr.Zero Then
'Set our ChildData
Dim cd As New WindowData()
With cd
'Set target class
.sWindowClass = ChildClass
'Set target index
.iWindowIndex = ChildIndex
'Zero current index
.iCurIndex = 0
'Zero Handle
.hWnd = IntPtr.Zero
End With
' Setup new enum
Dim myEnumChildProc As New EnumChildProc(AddressOf EnumChild)
' Enum childwindows
EnumChildWindows(ParentHandle, myEnumChildProc, cd)
'Return window handle
Return cd.hWnd
End If
'return Zero
Return IntPtr.Zero
End Function
Private Function GetChild(ByVal MainClass As String, ByVal MainCaption As String, ByVal ChildStyle As Integer) As IntPtr
'Get Handle Of Main Window
Dim iMain As IntPtr = FindMainWindow(MainClass, MainCaption)
'Only continue if Main window found
If iMain <> IntPtr.Zero Then
'Set our ChildData
Dim cd As New WindowData()
With cd
'Set target style
.iWindowStyle = ChildStyle
'Zero current index
.iCurIndex = 0
'Zero Handle
.hWnd = IntPtr.Zero
End With
' Setup new enum
Dim myEnumChildProc As New EnumChildProc(AddressOf EnumChildByStyle)
' Enum childwindows
EnumChildWindows(iMain, myEnumChildProc, cd)
'Return window handle
Return cd.hWnd
End If
'return Zero
Return IntPtr.Zero
End Function
Private Function GetChild(ByVal ParentHandle As IntPtr, ByVal ChildStyle As Integer) As IntPtr
'Only process if provided handle is not Zero
If ParentHandle <> IntPtr.Zero Then
'Set our ChildData
Dim cd As New WindowData()
With cd
'Set target style
.iWindowStyle = ChildStyle
'Zero current index
.iCurIndex = 0
'Zero Handle
.hWnd = IntPtr.Zero
End With
' Setup new enum
Dim myEnumChildProc As New EnumChildProc(AddressOf EnumChildByStyle)
' Enum childwindows
EnumChildWindows(ParentHandle, myEnumChildProc, cd)
'Return window handle
Return cd.hWnd
End If
'return Zero
Return IntPtr.Zero
End Function
'Call back for EnumChildWindows
Private Function EnumChild(ByVal hWnd As IntPtr, ByRef data As WindowData) As Int32
Dim ClassName As New StringBuilder(1024)
' Get the Child class name
GetClassName(hWnd, ClassName, ClassName.Capacity)
'Compair if its the class name we are looking for
'Use .Contains to allow partial Class match
'If ClassName.ToString().Contains(data.sWinClass) Then
'Use = to require perfect Class match
If ClassName.ToString() = data.sWindowClass Then
' Class found - Increase Current Index by 1
data.iCurIndex += 1
' Check if class is at correct index
If data.iWindowIndex = data.iCurIndex Then
data.hWnd = hWnd
'Stop Enum
Return 0
End If
End If
'Continue Enum
Return 1
End Function
'Call back for EnumChildWindows
Private Function EnumChildByStyle(ByVal hWnd As IntPtr, ByRef data As WindowData) As Int32
If data.iWindowStyle = GetWindowLong(hWnd, GWL_STYLE) Then
data.hWnd = hWnd
'Stop Enum
Return 0
End If
'Continue Enum
Return 1
End Function
Private Function FindMainWindow(ByVal wndclass As String, ByVal title As String) As IntPtr
If Not String.IsNullOrEmpty(wndclass) And Not String.IsNullOrEmpty(title) Then
Dim sd As New WindowData()
If String.IsNullOrEmpty(wndclass) Then
' Search by Caption only
'Set our SearchData
With sd
'Set Caption
.sWindowText = title
'Zero Handle
.hWnd = IntPtr.Zero
End With
'Enum top level windows
EnumWindows(New EnumWindowsProc(AddressOf EnumByCaption), sd)
ElseIf String.IsNullOrEmpty(title) Then
' Search by Class only
'Set our SearchData
With sd
'Set Class
.sWindowClass = wndclass
'Zero Handle
.hWnd = IntPtr.Zero
End With
'Enum top level windows
EnumWindows(New EnumWindowsProc(AddressOf EnumByClass), sd)
Else
' Search by Class and Caption
'Set our SearchData
With sd
'Set Class
.sWindowClass = wndclass
'Set Caption
.sWindowText = title
'Zero Handle
.hWnd = IntPtr.Zero
End With
'Enum top level windows
EnumWindows(New EnumWindowsProc(AddressOf EnumByClassAndCaption), sd)
End If
'Return Window Handle
Return sd.hWnd
Else
Return IntPtr.Zero
End If
End Function
Private Function EnumByClassAndCaption(ByVal hWnd As IntPtr, ByRef data As WindowData) As Boolean
' Check classname and title
' This is different from FindWindow() in that the code below allows partial matches
Dim sb As New StringBuilder(1024)
GetClassName(hWnd, sb, sb.Capacity)
'Use .Contains to allow partial Class match
'If sb.ToString().Contains(data.sWinClass) Then
'Use = to require perfect Class match
If sb.ToString() = data.sWindowClass Then
sb = New StringBuilder(1024)
GetWindowText(hWnd, sb, sb.Capacity)
'Use .Contains to allow partial Caption match
If sb.ToString().Contains(data.sWindowText) Then
'Use = to require perfect Caption match
'If sb.ToString() = data.sTitle Then
data.hWnd = hWnd
' Found the wnd, halt enumeration
Return False
End If
End If
' Continue Enum
Return True
End Function
' Return first window with matching class
Private Function EnumByClass(ByVal hWnd As IntPtr, ByRef data As WindowData) As Boolean
' Check classname and title
' This is different from FindWindow() in that the code below allows partial matches
Dim sb As New StringBuilder(1024)
GetClassName(hWnd, sb, sb.Capacity)
'Use .Contains to allow partial Class match
'If sb.ToString().Contains(data.sWinClass) Then
'Use = to require perfect Class match
If sb.ToString() = data.sWindowClass Then
data.hWnd = hWnd
' Found the wnd, halt enumeration
Return False
End If
' Continue Enum
Return True
End Function
' Return first window with matching caption
Private Function EnumByCaption(ByVal hWnd As IntPtr, ByRef data As WindowData) As Boolean
' Check classname and title
' This is different from FindWindow() in that the code below allows partial matches
Dim sb As New StringBuilder(1024)
GetWindowText(hWnd, sb, sb.Capacity)
'Use .Contains to allow partial Caption match
If sb.ToString().Contains(data.sWindowText) Then
'Use = to require perfect Caption match
'If sb.ToString() = data.sTitle Then
data.hWnd = hWnd
' Found the wnd, halt enumeration
Return False
End If
' Continue Enum
Return True
End Function
#End Region
Private Sub btnFind_MouseDown(sender As System.Object, e As System.Windows.Forms.MouseEventArgs) Handles btnFind.MouseDown
Cursor = Cursors.Cross
'Me.Visible = False
End Sub
Private Sub btnFind_MouseUp(sender As System.Object, e As System.Windows.Forms.MouseEventArgs) Handles btnFind.MouseUp
Cursor = Cursors.Default
'Me.Visible = True
Dim wd As WindowData = GetData()
If wd IsNot Nothing Then
If Not wd.hWnd = IntPtr.Zero Then
Dim result As Boolean = PostMessage(wd.hWnd, WM_LBUTTONDOWN, IntPtr.Zero, CType(&H10001, IntPtr))
result = PostMessage(wd.hWnd, WM_LBUTTONUP, IntPtr.Zero, CType(&H10001, IntPtr))
End If
End If
End Sub
End Class