Multivalued inputs are passed to the script as a pseudo-JSON string and can generally be parsed using the JSON.parse function. You might have noticed my hedging in that sentence... there are some gotchas... here are three and how to work around them:
1. If you have multiple input parameters, you need to separate them first
You can use a function like the following (from the help documentation and sample project):
function getArgumentMap(args) {
if (args == null) {
throw new Error('Invalid arguments.');
}
const argMap = {};
// eslint-disable-next-line no-plusplus
for (let i = 2; i < args.length; i++) {
const arg = args[i];
const idx = arg.indexOf('=');
if (idx > 0) {
const key = arg.substring(0, idx);
const val = arg.substring(idx + 1);
argMap[key] = val;
}
}
return argMap;
}
2. You need to remove a pair of wrapping single quotes
Something like this should work:
var inputs = getArgumentMap(process.argv);
var unqoutedList = inputs['List'].substr(1, inputs['List'].length - 2);
3. Individual string values within the multivalue input need to be wrapped in quotes
When testing in the editor:

In Workflow:
For example, I used an Assign Token Values activity to create a multivalued token and it need to look like this:
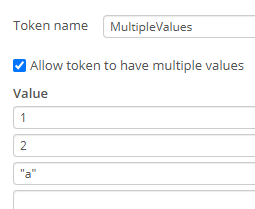
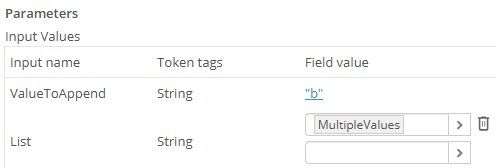