replied on October 17, 2023
Since you tagged this as Version 10, we know you are using the Classic Designer, and thus Javascript is going to be a valid option.
Here's an example of some code that populates an email into an email field that has class name of myEmailField based on the selections in a drop-down field that has class name of myDropdownField.
//Note that this code assumes that there is only a single field with the
//myDropdownField class name - it won't work as expected if there is more
//than one.
$(document).ready(function() {
//Make the department email field readonly. Doing it here instead of
//on the Layout page means it will allow changes via code. If it's
//readonly from the Layout page, the changes won't save.
$('.myEmailField input').attr('readonly', 'true');
//When the Department dropdown changes, populate the department email field.
$('.myDropdownField select').change(function() {
var dropdownSelect = $(this).val();
if (dropdownSelect == 'Accounting') {
$('.myEmailField input').val('accounting@fakeaddress.com');
}
else if (dropdownSelect == 'Finance') {
$('.myEmailField input').val('finance@fakeaddress.com');
}
else if (dropdownSelect == 'Marketing') {
$('.myEmailField input').val('marketing@fakeaddress.com');
}
else if (dropdownSelect == 'IT') {
$('.myEmailField input').val('it@fakeaddress.com');
}
else if (dropdownSelect == 'Management') {
$('.myEmailField input').val('management@fakeaddress.com');
}
});
});
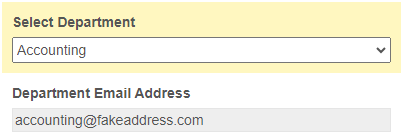
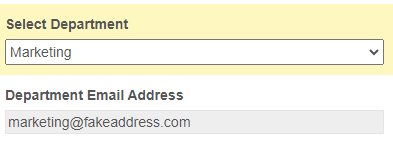
If you want to share specifics of the fields/options you are using and the results you need, I can provide a more tailored Javascript solution.