jquery.mask.min.js can no longer be used as new form designer doesn't use JQuery. You can write your own phoneMask function similar as following and call it when need:
function phoneMask (phone) {
return phone.replace(/\D/g, '')
.replace(/^(\d)/, '($1')
.replace(/^(\(\d{3})(\d)/, '$1) $2')
.replace(/(\d{3})(\d{1,5})/, '$1-$2')
.replace(/(-\d{4})\d+?$/, '$1');
}
LFForm.onFieldBlur(function(){ LFForm.setFieldValues({fieldId: 2}, phoneMask(LFForm.getFieldValues({fieldId: 2}))) }, {fieldId: 2})
You can also put some common used functions in a separate JS file and add the URL for the file in the external URL and call the functions from inline JavaScript.
Take the above one for example, you can put following pieces of code in the JS file and the URL to access the file is https://xxx.laserfiche.com/Forms/js/mask.js
function phoneMask (phone) {
return phone.replace(/\D/g, '')
.replace(/^(\d)/, '($1')
.replace(/^(\(\d{3})(\d)/, '$1) $2')
.replace(/(\d{3})(\d{1,5})/, '$1-$2')
.replace(/(-\d{4})\d+?$/, '$1');
}
You add the URL in the external tab of JavaScript for the form
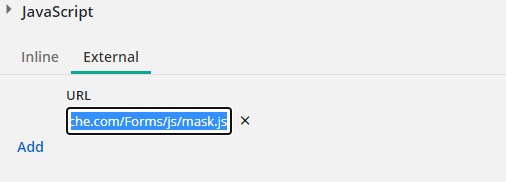
and call the function from Inline JavaScript with following piece of code:
LFForm.onFieldBlur(function(){ LFForm.setFieldValues({fieldId: 2}, phoneMask(LFForm.getFieldValues({fieldId: 2}))) }, {fieldId: 2})