I have a way to do it on the classic designer with Javascript.
This is tested on Forms 11 Update 2.
On your form, enable pagination. Make sure there is at least a first and last page. Then add as many pages as you want in between those pages. Here's an example with a maximum possible of 25. You can name the pages whatever you want:

There needs to be a limit because you have to add a page for each possible record. But all you are doing is adding the pages, you are not putting anything on the pages, so you can easily add quite a lot of them if needed. Important: Make sure the number on line 64 of the Javascript below is matching the number of pages - because my example has 25 page, the line of Javascript says 25. The line will help ensure rows are not added to the collection that don't have pages set-up for them.
On the First page (I named mine "Start"):
- Add a number field. I named mine "Number of Records".
Leave it Do not make it read-only. Give it these two CSS Class Names: javascriptReadOnly recordCount
- Add your collection. Give it the CSS Class Name of: pagesCollection
- Add as many fields as needed to your collection. Give one of the fields (only one) the CSS Class Name of: recordCounter
Add this CSS to your form:
/*Hide the option to delete rows from the collection because the code
to move them to different pages isn't set-up to handle deletions*/
.cf-collection-delete { display: none!important; }
/*Hide the Collection Title*/
.pagesCollection h2, .cf-section-header { display: none!important; }
/*Hide the border at the bottom of each collection row, otherwise
they are left behind on the first page*/
hr.end_set { display: none!important; }
Add this Javascript to your form:
$(document).ready(function () {
//Make the javascriptReadOnly fieldsread-only - this has to be done
//via Javascript, otherwise the field values cannot be populated
//via Javascript.
$('.javascriptReadOnly input').each(function() {
$(this).attr('readonly', 'true');
$(this).removeClass('hasDatepicker');
$(this).parent().find('.ui-datepicker-trigger').hide();
});
$('.javascriptReadOnly select').each(function() {
$(this).attr('readonly', 'true');
});
$('.javascriptReadOnly textarea').each(function() {
$(this).attr('readonly', 'true');
});
//When the form first loads - hide all of the pages except
//the first and last ones. They will be shown later as needed.
//This mimics the way the items are hidden via the Field Rules.
HideAllPages();
function HideAllPages() {
//Version of the code for the active form:
if($('.recordCount input').val() != undefined) {
$('.cf-page:not(:first):not(:last)').each(function() {
var pageID = $(this).attr('id');
$('.cf-pagination-tabs li[page=' + pageID + ']').addClass('hidden').attr('aria-hidden', 'true');
$('.cf-page[id=' + pageID + ']').addClass('hidden lf-container-hidden').attr('aria-hidden', 'true');
});
}
//Version of the code for the read-only form:
else {
$('.cf-page-readonly:not(:first):not(:last)').each(function() {
var pageID = $(this).attr('id');
$('.cf-page-readonly[id=' + pageID + ']').addClass('hidden lf-container-hidden');
});
}
}
//When the form first loads, and when new rows are added to the collection,
//cycle through each row and move it to a new page and unhide that page.
//Additionally, the count of records is updated.
MoveCollectionToPage();
$('.pagesCollection .cf-collection-append').click(MoveCollectionToPage);
function MoveCollectionToPage() {
//Version of the code for the active form:
if($('.recordCount input').val() != undefined) {
$('.pagesCollection .rpx').each(function() {
$('.cf-page.hidden:first').each(function() {
var pageID = $(this).attr('id');
$('.cf-pagination-tabs li[page=' + pageID + ']').removeClass('hidden').removeAttr('aria-hidden');
$('.cf-page[id=' + pageID + ']').removeClass('hidden lf-container-hidden').removeAttr('aria-hidden');
$('.pagesCollection .rpx:first').each(function() {
$('.cf-page[id=' + pageID + '] .cf-pagination-btn').before($(this));
});
});
});
var numberOfRecords = 0;
$('.recordCounter').each(function() {
numberOfRecords++;
});
$('.recordCount input').val(numberOfRecords);
//Hide the option to add more records if the count is at least 25
if(numberOfRecords >= 25) {
$('.pagesCollection .cf-collection-append').hide();
}
}
//Version of the code for the read-only form:
else {
$('.pagesCollection .rpx').each(function() {
$('.cf-page-readonly.hidden:first').each(function() {
var pageID = $(this).attr('id');
$('.cf-page-readonly[id=' + pageID + ']').removeClass('hidden lf-container-hidden');
$('.pagesCollection .rpx:first').each(function() {
$('.cf-page-readonly[id=' + pageID + '] h2').after($(this));
});
});
});
}
}
});
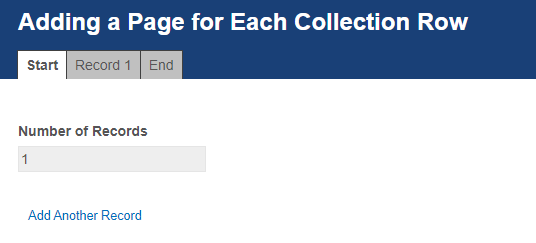
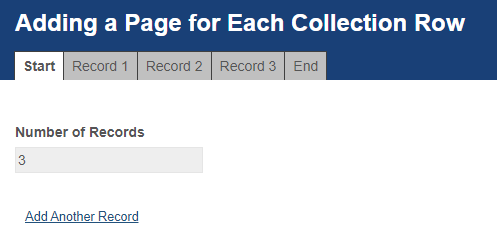
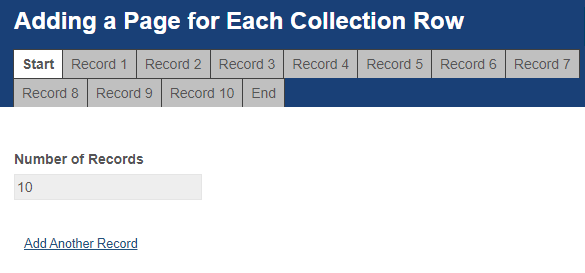