The structure of the field in the new designer is completely different than the structure of the field on the classic designer. The classic designer is more basic.
The downside, if they were to change the structure of the field on the classic designer, it could easily break scripts used by people all over.
The good news, is there are a lot of great resources out there to help make this work without Laserfiche needing to change the basic structure of the field in the classic designer.
I recommend downloading the Chosen JQuery plugin: https://github.com/harvesthq/chosen/releases
Put the files into your Server that is running LFForms at this location:
Then on your form, your drop-down field(s) should include CSS Class name of chosenSelect.
Then add this Javascript:
$(document).ready(function () {
//Set the dropdown field(s) with the chosenSelect class to use the
//functionality from the Chosen JQuery library.
//The if statement ensures it only processes on a live form and not
//the archival or read-only versions.
if($('.chosenSelect select').val() != undefined)
{
var serverAddress = 'https://SERVERNAME/forms'; //NEED TO LIST YOUR SERVER NAME HERE!
var scriptAddress = '/js/chosen_v1.8.7/chosen.jquery.js';
var styleAddress = '/js/chosen_v1.8.7/chosen.css';
$('head').append('<link rel="stylesheet" type="text/css" href="' + serverAddress + styleAddress + '">');
$.getScript(serverAddress + scriptAddress, function () {
$('.chosenSelect select').each(function() {
$(this).chosen();
});
});
}
});
When the form is loaded, the script loads in the Chosen stylesheet and Chosen script, and applies it all of your select fields that have the choseSelect class.
End result looks pretty good.

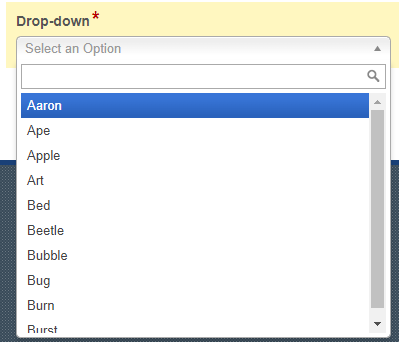
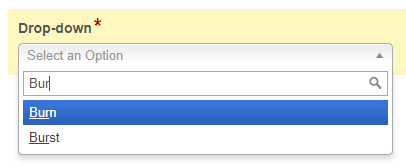
Really easy to implement in LFForms Classic Designer right now (note, I'm running Version 11 Update 2 for these examples).
*EDIT TO ADD* That script works on a drop-down that has the options set on the Layout Page. If instead you are loading the options from a lookup, things need to be tweaked slightly. For example, if the population of the field happens on form load, you can make it work by using
$(document).on("onloadlookupfinished",function(event) {
instead of
$(document).ready(function () {
like this:
$(document).on("onloadlookupfinished",function(event) {
//Set the dropdown field(s) with the chosenSelect class to use the
//functionality from the Chosen JQuery library.
//The if statement ensures it only processes on a live form and not
//the archival or read-only versions.
if($('.chosenSelect select').val() != undefined)
{
var serverAddress = 'https://SERVERNAME/forms'; //NEED TO LIST YOUR SERVER NAME HERE!
var scriptAddress = '/js/chosen_v1.8.7/chosen.jquery.js';
var styleAddress = '/js/chosen_v1.8.7/chosen.css';
$('head').append('<link rel="stylesheet" type="text/css" href="' + serverAddress + styleAddress + '">');
$.getScript(serverAddress + scriptAddress, function () {
$('.chosenSelect select').each(function() {
$(this).chosen();
});
});
}
});
*EDIT TO ADD - AGAIN* Here is the updated script that I posted in another comment. This one is more robust about handling the field whether it is populated via the Layout Page or via Lookups, and can also handle the field being hidden initially by Field Rules - if, in addition to putting the chosenSelect class on your dropdowns, you also put the chosenTrigger class on whatever field(s) is/are triggering your field rules - any time any field with the chosenTrigger class is changed, the chosenSelect fields are refreshed. The only part I don't have working is that if the chosenSelect field is used to trigger a Lookup, that isn't working - the change event fires, but the event to trigger the lookup attempt doesn't, and I'm not certain why:
$(document).ready(function () {
//Set the dropdown field(s) with the chosenSelect class to use the
//functionality from the Chosen JQuery library.
//The if statement ensures it only processes on a live form and not
//the archival or read-only versions.
if($('.chosenSelect select').val() != undefined)
{
var serverAddress = 'http://SERVERNAME/forms'; //NEED TO LIST YOUR SERVER NAME HERE!
var scriptAddress = '/js/chosen_v1.8.7/chosen.jquery.js';
var styleAddress = '/js/chosen_v1.8.7/chosen.css';
$('head').append('<link rel="stylesheet" type="text/css" href="' + serverAddress + styleAddress + '">');
$.getScript(serverAddress + scriptAddress, function () {
$('.chosenSelect select').each(function() {
$(this).chosen();
});
});
}
//When any field that has the chosenTrigger class changes, check
//for select fields that have been unhidden and need the chosen
//script applied to them.
$('.chosenTrigger').change(function(){
$('.chosenSelect select').each(function() {
$(this).trigger('chosen:updated');
$(this).parent().find('.chosen-container').css('width', $(this).css('width'));
});
});
});
//Update the dropdowns with the Chosen script when lookups are complete
$(document).on("onloadlookupfinished",function(event) {
$('.chosenSelect select').each(function() {
$(this).trigger('chosen:updated');
});
});
//Update the dropdowns with the Chosen script when lookups are complete
$(document).on("lookupcomplete",function(event) {
$('.chosenSelect select').each(function() {
$(this).trigger('chosen:updated');
});
});
*EDIT TO ADD - THIRD TIME!* Here's modified code that works to trigger the lookup when one of the chosen fields is the source of the lookup:
$(document).ready(function () {
//Set the dropdown field(s) with the chosenSelect class to use the
//functionality from the Chosen JQuery library.
//The if statement ensures it only processes on a live form and not
//the archival or read-only versions.
if($('.chosenSelect select').val() != undefined)
{
var serverAddress = 'https://SERVERNAME/forms'; //NEED TO LIST YOUR SERVER NAME HERE!
var scriptAddress = '/js/chosen_v1.8.7/chosen.jquery.js';
var styleAddress = '/js/chosen_v1.8.7/chosen.css';
$('head').append('<link rel="stylesheet" type="text/css" href="' + serverAddress + styleAddress + '">');
$.getScript(serverAddress + scriptAddress, function () {
$('.chosenSelect select').each(function() {
$(this).chosen();
});
});
}
//When any field that has the chosenTrigger class changes, check
//for select fields that have been unhidden and need the chosen
//script applied to them.
$('.chosenTrigger').change(function(){
$('.chosenSelect select').each(function() {
$(this).trigger('chosen:updated');
$(this).parent().find('.chosen-container').css('width', $(this).css('width'));
});
});
//Because the chosen script appears to be preventing the lookups
//from being triggered by the changes to fields, this watches
//for changes to the field and manually triggers the lookup event.
$('.chosenSelect select').change(function() {
$(this).trigger('lookup');
});
});
//Update the dropdowns with the Chosen script when lookups are complete
$(document).on("onloadlookupfinished",function(event) {
$('.chosenSelect select').each(function() {
$(this).trigger('chosen:updated');
});
});
//Update the dropdowns with the Chosen script when lookups are complete
$(document).on("lookupcomplete",function(event) {
$('.chosenSelect select').each(function() {
$(this).trigger('chosen:updated');
});
});