Instead of using JavaScript to hide/show the button, I always use the following approach:
1) Use CSS to hide the built-in Submit button
.Submit {
display: none !important;
}
2) Add a custom HTML element with my own button
<button id="submitBtn" type="button">Submit</button>
(You can add CSS to style it however you choose)
#submitBtn {
width: 100px;
height: 30px;
color: #000000;
border-color: #404040;
border-width: 1px;
border-style: solid;
background: #00ff00;
}
3) Add JavaScript to tie the custom button to the default one
$(document).ready(function(){
$('#submitBtn').on('click', function(){
$('.Submit').click();
});
});
4) Use Field Rules to show/hide your custom button
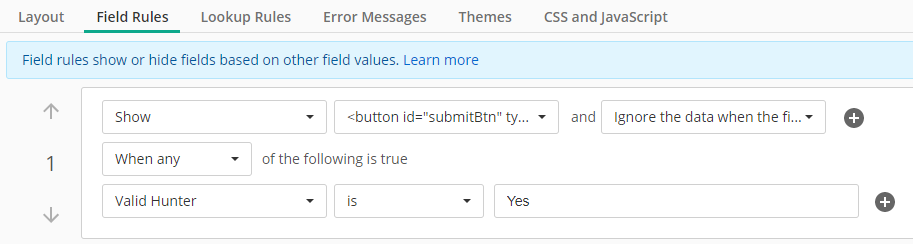
However, since you're dealing with a collection, you'll want to flip the condition so it Hides the button when any fields are not equal to Yes (i.e., only show it when they're ALL Yes).
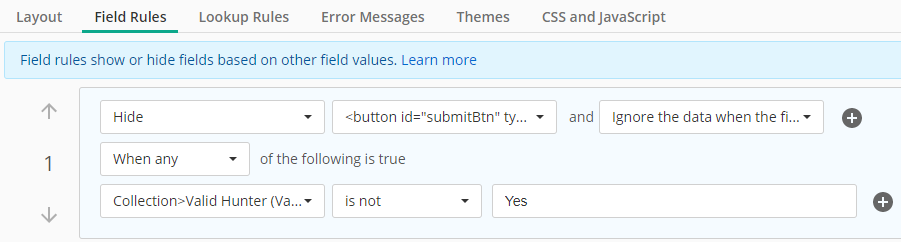
For the sake of being thorough, there's a few reasons why your current code wouldn't work:
- Custom event handlers are attached when the page loads, so any collection groups added after that won't have those handlers. To get around this, you need to use event delegation.
- The $('.hideSubmit') selector has a couple of issues. First, it is selecting the parent container, not the input within the parent. Second, there's nothing in there to tell it which one to select since there could be many in the collection.
- Because your condition is only checking one value, if even one of your target fields has a Yes value, it would still show the button when the other values may not be valid
For the first two issues, you can check out the following post to see some examples of event delegation and how to reference the "sibling" fields in each collection group.
Table and Collection JavaScript Event Handlers - Laserfiche Answers
For the last item, you'd need to check every single "Valid Hunter" field each time to make sure they are all yes before deciding whether to hide or show (this is part of the reason using the custom html button and field rules is more manageable).