replied on February 18, 2021
I know this is not exactly what you want, but here's a workaround, that allows you to add textboxes to your document using an SDK Script activity and some tokens. You can modify the token values and run the same script again to add more textboxes.
This gives you a little more direct control over the different specifics of the textbox and does appear to be using top-left positioning instead of center positioning - so it's a little more intuitive.
Here's the tokens that are needed:
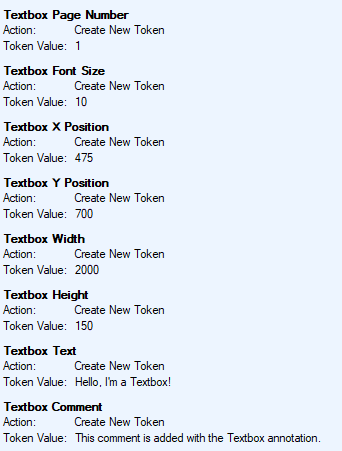
And here's the script to include in the SDK Script activity (this assumes Workflow version 10.4, you need to edit line 15 if you are on a different version).
namespace WorkflowActivity.Scripting.SDKScript
{
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Data.SqlClient;
using System.Text;
using Laserfiche.RepositoryAccess;
using Laserfiche.RepositoryAccess.Common;
/// <summary>
/// Provides one or more methods that can be run when the workflow scripting activity is performed.
/// </summary>
public class Script1 : RAScriptClass104
{
/// <summary>
/// This method is run when the activity is performed.
/// </summary>
protected override void Execute()
{
//Make sure that we are working with a document...
if(this.BoundEntryInfo.EntryType == EntryType.Document)
{
//Retrieve all Token values from the Workflow
int pToken = Convert.ToInt32(this.GetTokenValue("Textbox Page Number"));
int xToken = Convert.ToInt32(this.GetTokenValue("Textbox X Position"));
int yToken = Convert.ToInt32(this.GetTokenValue("Textbox Y Position"));
int wToken = Convert.ToInt32(this.GetTokenValue("Textbox Width"));
int hToken = Convert.ToInt32(this.GetTokenValue("Textbox Height"));
int fToken = Convert.ToInt32(this.GetTokenValue("Textbox Font Size"));
string tToken = this.GetTokenValue("Textbox Text").ToString();
string cToken = this.GetTokenValue("Textbox Comment").ToString();
//Get a reference to page 1 of the entry...
DocumentInfo di = Document.GetDocumentInfo(this.BoundEntryId, this.RASession);
PageInfo pi = di.GetPageInfo(pToken);
//Create a new TextBoxAnnotation...
TextBoxAnnotation textBox = new TextBoxAnnotation();
//Set the coordinates and other properties from the token values...
LfPoint textBoxPosition = new LfPoint(xToken, yToken);
LfSize textBoxSize = new LfSize(wToken, hToken);
textBox.Coordinates = new LfRectangle(textBoxPosition, textBoxSize);
textBox.TextSize = fToken;
textBox.BorderColor = LfColor.TRANSPARENT;
textBox.FillColor = LfColor.TRANSPARENT;
textBox.Text = tToken;
textBox.Comment = cToken;
//Add the annotation to the page...
pi.AddAnnotation(textBox);
}
}
}
}