Fortunately Forms imports moment.js by default, which makes working with date and time values WAY easier than it is with vanilla JavaScript and JQuery.
I set a custom CSS selector on the table ("timeSheet") and additional custom CSS selectors on the time in and time out fields ("timeIn" and "timeOut" respectively in my example).
$(document).ready(function(){
// trigger when time in changes on any row of table
$('.timeSheet').on('change','.timeIn',function(){
// get time value and am/pm value
var t1 = $(this).find('input').val();
var p1 = $(this).find('select').val();
// convert values to moment.js time object
var time = moment.utc(t1 + p1,'h:mm:ss a');
// add 9 hours
time.add(9, 'hours');
// get new time and am/pm values
// need to use lowercase am/pm to match actual value attributes of am/pm options
var t2 = time.format('h:mm:ss');
var p2 = time.format('a');
// find time out field for current row
var timeOut = $(this).parent('tr').find('.timeOut');
// set time and am/pm values
timeOut.find('input').val(t2).change();
timeOut.find('select').val(p2).change();
});
});
This code can certainly be condensed into far fewer lines of code, but I kept it in a "one step at a time" format to make it easier to understand what is being done.
Of course if you switched to 24H time or something you would need to change the formatting, but this worked for h:mm:ss am/pm like you had in your screenshot.
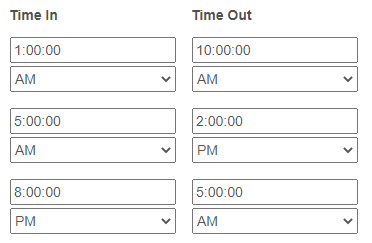
One thing to note however is that changes made with JavaScript won't be saved if your field is read-only when the change occurs, so if you plan to make "time out" a read only field you'll need a couple extra lines of code.
Here is a link to a moment.js cheatsheet that explains the formatting I used.
https://devhints.io/moment