From the original post, it sounded like you wished to highlight either a single cell or any cell with a value of -1. In our email exchange, I found that you actually want to highlight any row where the first cell/column has a value of -1. You also expressed that you wanted to get all cells in the row no matter how many cells. So here is what I think will server your purposes.
Start by downloading and installing Open XML SDK 2.5 for Microsoft Office (Note: it installs into Program Files (x86), but will still work with Workflow x64)
Next, add a SDK Script Activity to your workflow and set the Script's Default Entry to the laserfiche entry with the work document,
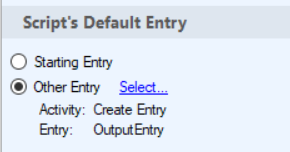
Then open the code block for editing. First you need to add the references for Laserfiche.DocumentServices and the OpenXML SDK. In the Project Explorer pane, right click "References" and click "Add Reference..."
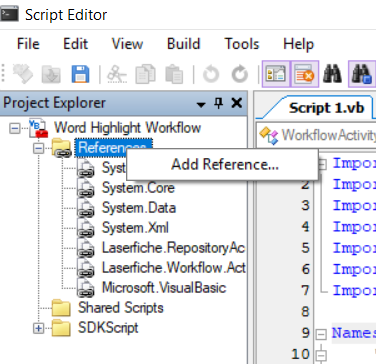
In the resulting Dialog window, in the search bar, type "open" and then after selecting "DocumentFormat.OpenXml", click "OK".
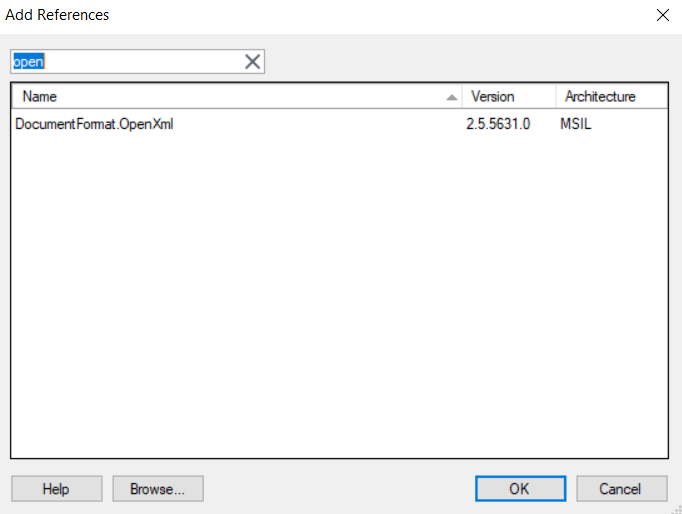
Next check the version of "Laserfiche.RepositoryAccess" by right clicking it and selecting "Details".
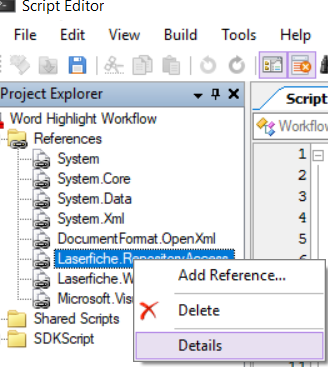
Once the details are shown, it will include the .NET version followed by an Underscore and then the LF RA version. When selecting DocumentServices, select the one that matches the RA.
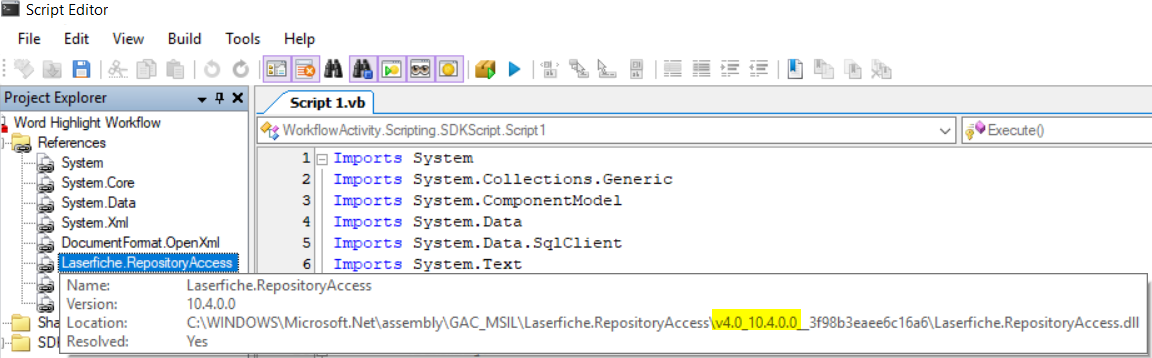
Now right click "References" and click "Add Reference..." again. In the resulting Dialog window, in the search bar, type "laserfiche.doc" and then after selecting the DocumentServices version that matches the RA, click "OK".
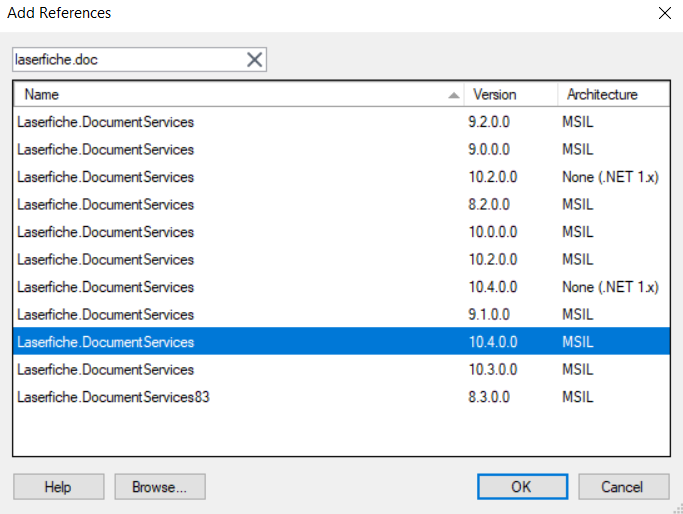
Now that the references are added, add some import statements to make the reference files readily available in the code. At the top of the code window, will be a list of Import statements. Add the following 3 statements
Imports Laserfiche.DocumentServices
Imports DocumentFormat.OpenXml
Imports DocumentFormat.OpenXml.Packaging
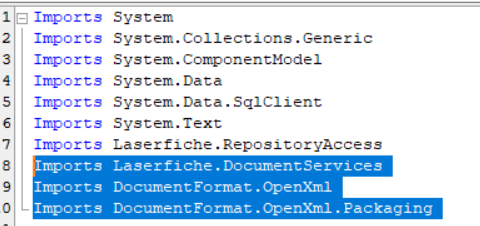
Finally the references are added and it is time to update the code. Between the line of code that has "Protected Overrides Sub Execute()" and the line that has "End Sub", paste the following code:
'Write your code here. The BoundEntryInfo property will access the entry, RASession will get the Repository Access session
Dim sTempPath As String = "C:\Temp"
Dim sExpDocName As String = Nothing
Try
' Open LF Doc for Export
Using DocInfo As DocumentInfo = TryCast(BoundEntryInfo, DocumentInfo)
' Ensure LF Doc is not Nothing
If DocInfo IsNot Nothing Then
' Set Word Doc Name
sExpDocName = DocInfo.Name & ".docx"
' Create Exporter
Dim de As DocumentExporter = New DocumentExporter()
' Export LF Doc To Temp File
de.ExportElecDoc(DocInfo, System.IO.Path.Combine(sTempPath, sExpDocName))
' Open Temp .docx file
Using wordDoc As WordprocessingDocument = WordprocessingDocument.Open(System.IO.Path.Combine(sTempPath, sExpDocName), True)
' Get an enumerator of all Tables in Document
Dim enumTables As IEnumerable(Of Wordprocessing.Table) = wordDoc.MainDocumentPart.Document.Body.Elements(Of Wordprocessing.Table)
' Process each Table in document
For Each tbl As Wordprocessing.Table In enumTables
' Get an enumerator of all Rows in Table
Dim enumRows As IEnumerable(Of Wordprocessing.TableRow) = tbl.Elements(Of Wordprocessing.TableRow)
' Process each Row in Table
For Each tblRow As Wordprocessing.TableRow In enumRows
' Get First Cell in Row
Dim FirstCell As Wordprocessing.TableCell = tblRow.GetFirstChild(Of Wordprocessing.TableCell)
' Get an enumerator of all Cells in Row
Dim enumCells As IEnumerable(Of Wordprocessing.TableCell) = tblRow.Elements(Of Wordprocessing.TableCell)
' Process each Cell in Row
For Each tblCell As Wordprocessing.TableCell In enumCells
' Get Shading Property from Cell
Dim tblCellShading As Wordprocessing.Shading = tblCell.TableCellProperties.Shading
' Evaluate Shading Property
If tblCellShading Is Nothing Then
' Check First Cell for processing
If FirstCell.InnerText = "-1" Then
' Create new Shading Property
Dim NewShading As Wordprocessing.Shading = New Wordprocessing.Shading()
' Set Shading Property settings
NewShading.Color = "auto"
NewShading.Fill = "FFFF00" ' Yellow
NewShading.Val = Wordprocessing.ShadingPatternValues.Clear
' Add Shading Property
tblCell.TableCellProperties.AppendChild(Of Wordprocessing.Shading)(NewShading)
End If
Else
' Check First Cell for processing
If FirstCell.InnerText = "-1" Then
' Only modify if Shading is not Yellow
If tblCellShading.Fill <> "FFFF00" Then ' Yellow then
' Remove Existing Shading
tblCellShading.Remove()
' Create new Shading Property
Dim NewShading As Wordprocessing.Shading = New Wordprocessing.Shading()
' Set Shading Property settings
NewShading.Color = "auto"
NewShading.Fill = "FFFF00" ' Yellow
NewShading.Val = Wordprocessing.ShadingPatternValues.Clear
' Add Shading Property
tblCell.TableCellProperties.AppendChild(Of Wordprocessing.Shading)(NewShading)
End If
Else
' Remove Existing Shading
tblCellShading.Remove()
End If
End If
Next
Next
Next
' Close and Save Temp .docx file
End Using
End If
' Close LF Doc For Export
End Using
'Check for Temp Doc
Dim fiWordDoc As System.IO.FileInfo = New System.IO.FileInfo(System.IO.Path.Combine(sTempPath, sExpDocName))
If fiWordDoc.Exists Then
' Open LF Doc for Import
Using UpdateDocInfo As DocumentInfo = TryCast(BoundEntryInfo, DocumentInfo)
' Ensure LF Doc is not Nothing
If UpdateDocInfo IsNot Nothing Then
' Create Importer
Dim di As DocumentImporter = New DocumentImporter()
' Set Importer to import into LF Doc
di.Document = UpdateDocInfo
' Import Temp .docx file into LF Doc
di.ImportEdoc("application/vnd.openxmlformats-officedocument.wordprocessingml.document", System.IO.Path.Combine(sTempPath, sExpDocName))
' Set LF Doc File Extension
UpdateDocInfo.Extension = "docx"
' Save the Changes to the LF Doc
UpdateDocInfo.Save()
End If
' Close LF Doc For Import
End Using
End If
Catch ex As Exception
WorkflowApi.TrackError(ex.Message)
End Try
' Cleanup Temp .docx file
Dim fi As System.IO.FileInfo = New System.IO.FileInfo(System.IO.Path.Combine(sTempPath, sExpDocName))
If fi.Exists Then
fi.Delete()
End If
There is only 1 line of code to change and it is the first Dim Statement.
In the "sTempPath", set "C:\Temp" to a folder where the Workflow Service user can create, modify, and delete the temp word document.
The resulting Word doc will highlight each row that has a -1 in the first cell.
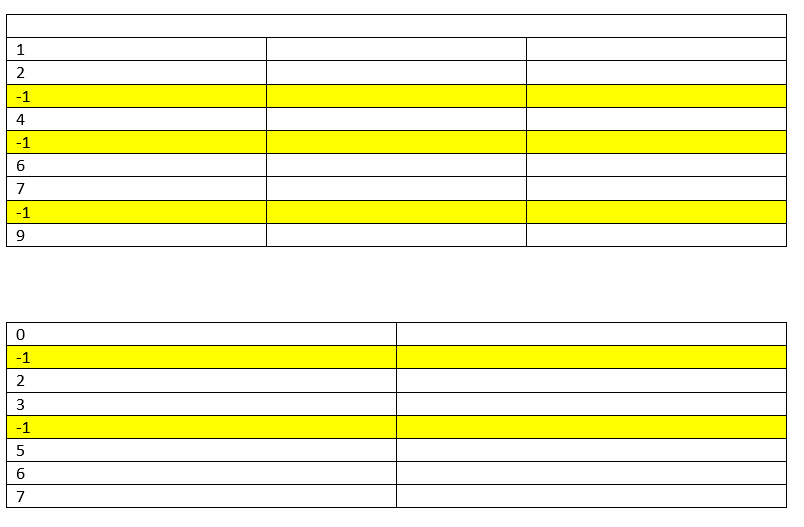