I use workflow to generate the excel document after form submission. I use tokens in my workflow to create the csv and then create the row data from form submission data.
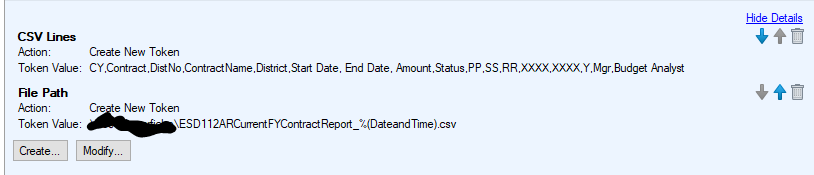
Then you need to create the file with the following script
namespace WorkflowActivity.Scripting.CSVFileCreation0
{
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Data.SqlClient;
using System.Text;
using Laserfiche.RepositoryAccess;
/// <summary>
/// Provides one or more methods that can be run when the workflow scripting activity is performed.
/// </summary>
public class Script1 : RAScriptClass102
{
private const string CSV_LINES_TOKEN = "%(CSV Lines#[]#)";
private const string FILE_PATH_TOKEN = "%(File Path)";
/// <summary>
/// This method is run when the activity is performed.
/// </summary>
protected override void Execute()
{
// Write your code here. The BoundEntryInfo property will access the entry, RASession will get the Repository Access session
var filePath = this.ReplaceTokensInString(FILE_PATH_TOKEN, true);
var csvText = this.ReplaceTokensInString(CSV_LINES_TOKEN, true) + "\r\n";
var csvData = System.Text.Encoding.UTF8.GetBytes(csvText);
using (System.IO.FileStream file = System.IO.File.Create(filePath)) {
file.Write(csvData, 0, csvData.Length);
}
}
}
}