Here is what I use:
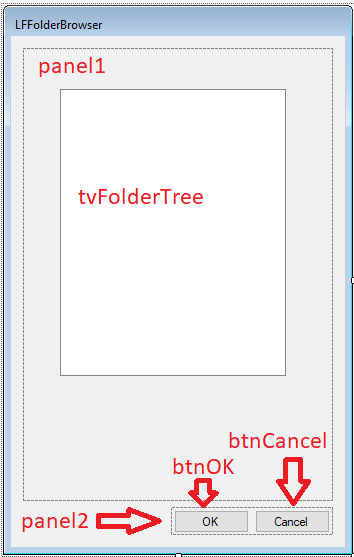
I add a 2nd form to the project and add 2 panels, 2 buttons, 1 Treeview, and 1 ImageList (not shown). To the ImageList, I add an open folder icon and then a folder icon and I point the TreeView ImageList property to the added ImageList.
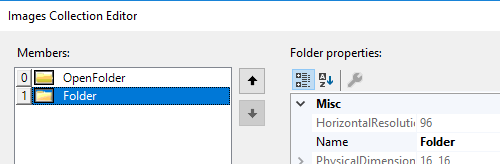
Here is the code for the folder browser:
public partial class LFFolderBrowser : Form
{
#region Property definition
// *********************************
// *********************************
// **Public Properties for Browser**
// *********************************
// *********************************
private int _EntryId;
public int EntryId
{
get
{
return _EntryId;
}
}
private string _Path;
public string Path
{
get
{
return _Path;
}
}
// **********************************
// **********************************
// **Private Properties for Browser**
// **********************************
// **********************************
private Session _RASession = null;
private Session RASession
{
get
{
return _RASession;
}
set
{
_RASession = value;
}
}
#endregion
#region LF Log In/Out definition
private void LFLogout()
{
// Only process if LFDatabase object is not nothing
if ((RASession != null))
{
try
{
// Log Out
RASession.LogOut();
RASession.Discard();
}
catch (Exception ex)
{
// Log Errors Here
}
// Ensure LFDatabse object is nothing
RASession = null;
}
}
private void LFLogin(string sServer, string sRepo, bool bWinAuth, bool bSSL, int SSLPort, string sUser = "admin", string sPW = "")
{
// Only process if LFDatabase object is nothing
if (RASession == null)
{
try
{
// Create LFServer object for server
Server lfserv = new Server(sServer);
// Set LFRepository object
RepositoryRegistration LFRepo = new RepositoryRegistration(lfserv, sRepo);
RASession = new Session();
RASession.ApplicationName = Application.ProductName;
// Process Authentication
if (bWinAuth)
{
// Use blank User and Password for WinAuth
RASession.LogIn(LFRepo);
}
else
{
//Do not use empty user name for LF Auth (if that is what was passed)
if (string.IsNullOrEmpty(sUser))
{
// Try using admin with blank password
sUser = "admin";
sPW = "";
}
RASession.LogIn(sUser, sPW, LFRepo);
}
}
catch (Exception ex)
{
// Log Errors Here
MessageBox.Show(ex.Message);
// Ensure Session object is nothing
RASession = null;
}
}
}
private void CloudLogin(string customerId, string username, string password)
{
if (RASession == null)
{
CloudTicket ticket = getCloudTicket(customerId, username, password);
if (ticket != null)
{
string repositoryName = getCloudRepositoryName(ticket) + ".laserfiche.com";
RepositoryRegistration repoReg = new RepositoryRegistration(repositoryName, repositoryName, 80, 443);
RASession = new Session();
RASession.IsSecure = true;
RASession.LogIn(username, password, repoReg);
}
}
}
private CloudTicket getCloudTicket(string customerId, string username, string password)
{
CloudTicket CurrentTicket = null;
try
{
CloudTicketRequestSettings ctrSettings = new CloudTicketRequestSettings();
ctrSettings.AccountId = customerId;
ctrSettings.UserName = username;
ctrSettings.Password = password;
CurrentTicket = CloudTicket.GetTicket(ctrSettings);
}
catch (Exception ex)
{
CurrentTicket = null;
}
return CurrentTicket;
}
private string getCloudRepositoryName(CloudTicket ticket)
{
string samlTokenXml = ticket.GetSamlToken();
string repositoryName = "";
using (XmlReader reader = XmlReader.Create(new StringReader(samlTokenXml)))
{
while (reader.Read())
{
if (reader.LocalName == "Attribute" && reader.GetAttribute("Name") == "http://laserfiche.com/identity/claims/catalyst/roles")
{
int depth = reader.Depth;
while (reader.Read() && reader.Depth > depth)
{
if (reader.NodeType == XmlNodeType.Element && reader.LocalName == "AttributeValue")
{
reader.Read();
string roleValue = reader.Value;
if (!string.IsNullOrEmpty(roleValue) && roleValue.Contains(":"))
{
int colonPos = roleValue.IndexOf(":");
string roleDomain = roleValue.Substring(0, colonPos);
string role = roleValue.Substring(colonPos + 1);
if (role == "CreateSession")
repositoryName = roleDomain;
}
}
}
}
}
}
return repositoryName;
}
#endregion
// Create new Browser with Cloud Login
public LFFolderBrowser(string customerId, string username, string password)
{
InitializeComponent();
CloudLogin(customerId, username, password);
if (RASession == null)
{
MessageBox.Show("Log in failed. Please correct problem and try again.");
this.Dispose();
}
}
// Create new Browser with On Prem Login
public LFFolderBrowser(string sServer, string sRepo, bool bWinAuth, bool bSSL, int SSLPort, string sUser = "admin", string sPW = "")
{
InitializeComponent();
LFLogin(sServer, sRepo, bWinAuth, bSSL, SSLPort, sUser, sPW);
if (RASession == null)
{
MessageBox.Show("Log in failed. Please correct problem and try again.");
this.Dispose();
}
}
private void LFFolderBrowser_Load(object sender, EventArgs e)
{
// Dock treeview into panel
tvFolderTree.Dock = DockStyle.Fill;
// Make sure there is a connection to the repository
if (RASession == null)
{
// No connection
MessageBox.Show("Log in failed. Please correct problem and try again.");
this.DialogResult = System.Windows.Forms.DialogResult.Cancel;
this.Close();
}
else
{
// Create new Node
TreeNode RootTreeNode = new TreeNode();
// Set the treeview imagekey
RootTreeNode.ImageKey = "Folder";
// Set Node Text
RootTreeNode.Text = RASession.Repository.Name;
// Make new Node expandable
RootTreeNode.Nodes.Add("");
// Add new Node to TreeView
tvFolderTree.Nodes.Add(RootTreeNode);
// Expand new Node
tvFolderTree.Nodes[0].Expand();
}
}
private void LFFolderBrowser_FormClosed(object sender, FormClosedEventArgs e)
{
LFLogout();
}
private void tvFolderTree_BeforeExpand(object sender, TreeViewCancelEventArgs e)
{
// Clear any existing child nodes
e.Node.Nodes.Clear();
// Get full path from node
string sFullPath = e.Node.FullPath;
// Remove repository name from path for LF use
if (sFullPath.Contains("\\"))
{
sFullPath = sFullPath.Replace(sFullPath.Substring(0, sFullPath.IndexOf("\\")), "");
}
else
{
sFullPath = "\\";
}
try
{
// Create FolderInfo object fron Node path
using (FolderInfo LFFolder = Folder.GetFolderInfo(sFullPath, RASession))
{
// configure which columns to retrieve
EntryListingSettings entrySetting = new EntryListingSettings();
// Only get folders
entrySetting.EntryFilter = EntryTypeFilter.Folders;
// Include Entry Type
entrySetting.AddColumn(SystemColumn.EntryType);
// Include Entry Name
entrySetting.AddColumn(SystemColumn.DisplayName);
// get the contents of the lf folder
using (FolderListing listing = LFFolder.OpenFolderListing(entrySetting, 1000))
{
// the listing is 1-based,
int rowCount = listing.RowsCount;
for (int i = 1; i <= rowCount; i++)
{
// Process only folders
// Listing should never get anything other than folder, but will check anyway
if (listing.GetDatumAsString(i, SystemColumn.EntryType).ToLower() == "folder")
{
// Create new Node
TreeNode NewTreeNode = new TreeNode();
// Set the treeview imagekey
NewTreeNode.ImageKey = "Folder";
// Set Node Text = folder name
NewTreeNode.Text = listing.GetDatumAsString(i, SystemColumn.DisplayName);
// Make new Node expandable
NewTreeNode.Nodes.Add("");
// Add new Node to TreeView
e.Node.Nodes.Add(NewTreeNode);
}
}
}
}
}
catch (Exception ex)
{
// Log Errors here
}
}
private void tvFolderTree_AfterSelect(object sender, TreeViewEventArgs e)
{
// Get full path from node
string sFullPath = e.Node.FullPath;
// Remove repository name from path for LF use
if (sFullPath.Contains("\\"))
{
sFullPath = sFullPath.Replace(sFullPath.Substring(0, sFullPath.IndexOf("\\")), "");
}
else
{
sFullPath = "\\";
}
try
{
// Create FolderInfo object fron Node path
using (FolderInfo LFFolder = Folder.GetFolderInfo(sFullPath, RASession))
{
// Get Entry ID of selected Node
_EntryId = LFFolder.Id;
// Get full path of folder
_Path = LFFolder.Path;
}
}
catch (Exception ex)
{
// Log errors here
}
}
private void btnOK_Click(object sender, EventArgs e)
{
if (string.IsNullOrEmpty(_Path))
{
_Path = "\\";
}
this.DialogResult = System.Windows.Forms.DialogResult.OK;
this.Close();
}
private void btnCancel_Click(object sender, EventArgs e)
{
this.DialogResult = System.Windows.Forms.DialogResult.Cancel;
this.Close();
}
}
On my main form, I have a Textbox for the LF folder path and a button to open the LF Folder Browser form.

The code I use in the picker button is:
private void btnLFFolderPicker_Click(object sender, EventArgs e)
{
// iLoginType Values
// 1 = Cloud
// 0 = On Prem
switch (iLoginType)
{
case 1:
LFFolderBrowser lfbCloud = new LFFolderBrowser(sCloudAccountID, sCloudUserName, sCloudPassword);
if (lfbCloud.IsDisposed == false)
{
if (lfbCloud.ShowDialog() == DialogResult.OK)
{
tbLFPath.Text = lfbCloud.Path;
}
if (lfbCloud.IsDisposed == false)
{
lfbCloud.Dispose();
}
}
break;
default:
LFFolderBrowser lfb = new LFFolderBrowser(sServerName, sRepositoryName, bWinAuth, bUseSSL, iSSLPort, sUserName, sPassword);
if (lfb.IsDisposed == false)
{
if (lfb.ShowDialog() == DialogResult.OK)
{
tbLFPath.Text = lfb.Path;
}
if (lfb.IsDisposed == false)
{
lfb.Dispose();
}
}
break;
}