Hi Peter,
That is a great idea!! I haven't implemented something like that but I might - instead of a chat I played with a secondary form (let's call it Contact Form) that will email our IT's department ticket system and automatically make a ticket (if you want to get fancy, you can even add a workflow on the Contact Form that will escalate it after certain time, depending on urgency).
Let's pretend that this is your form and the top you have the option to Contact the IT department (or really whoever):
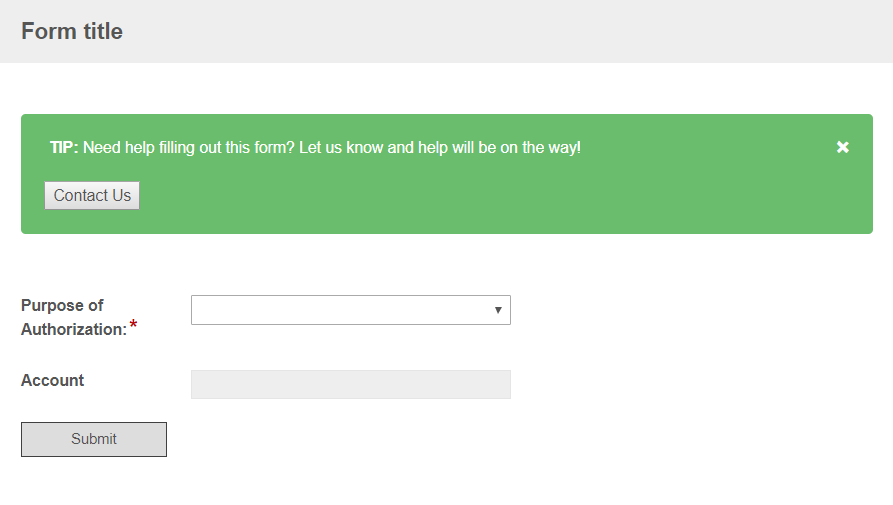
Then they can click on "Contact Us" button that will show the embedded Contact Form in an iframe. This gives you all kinds of creative possibilities again - you can disable the main Submit button when the Contact Form is submitted and display instructions in the window, or even redirect the entire page elsewhere - really depends of your need.
So this is what the Contact Form looks like when open:
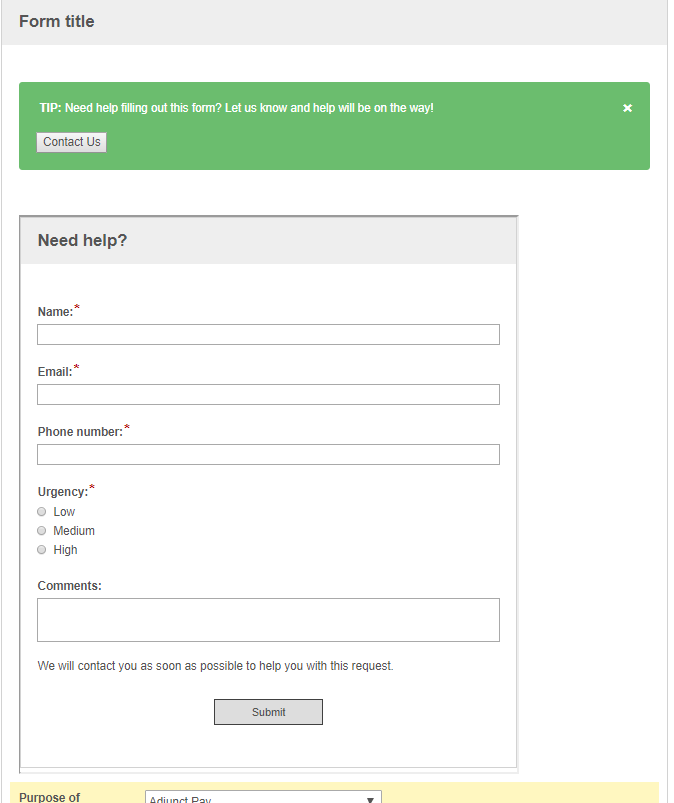
My code is setup to hide the main Submit button after they open the Contact Form and right now I didn't add a way to close it but that is doable as well.
The main form setup is fairly simple:
Add two HTML fields.
1st one is your alert window (in this case form section!) with the "Contact Us" button.
<div class="alert">
<span class="closebtn" onclick="this.parentElement.style.display='none';">×</span>
<b>TIP:</b> Need help filling out this form? Let us know and help will be on the way!
<br><br>
<input type="button" name="answer" value="Contact Us" id="clickMe">
</div>
2nd HTML field will automatically be hidden after click to be Done with Editing since we want it to be hidden by default:
<div id="contact" style="display:none;">
<br>
<iframe src="URLtoYourContactForm#inside" width="600px" height="670px" align="center"></iframe>
</div>
Now the CSS :
.alert {
padding: 20px;
background-color: #6BBD6E; /* Green */
color: white;
margin-bottom: 15px;
}
/* The close button */
.closebtn {
margin-left: 15px;
color: white;
font-weight: bold;
float: right;
font-size: 22px;
line-height: 20px;
cursor: pointer;
transition: 0.3s;
}
.closebtnForm {
margin-left: 15px;
color: white;
font-weight: bold;
float: right;
font-size: 22px;
line-height: 20px;
cursor: pointer;
transition: 0.3s;
}
/* When moving the mouse over the close button */
.closebtn:hover {
color: black;
}
Javascript:
$( document ).ready(function() {
//Displays the iframe form
function showDiv() {
document.getElementById('contact').style.display = "block";
//hides the submit button
$(".action-btn.Submit").hide();
}
//Makes the "Contact Us" button run the showDIV function
var el = document.getElementById("clickMe");
if (el.addEventListener)
el.addEventListener("click", showDiv, false);
else if (el.attachEvent)
el.attachEvent('onclick', showDiv);
});
Let me know if this helps, I know that it is not exactly what you needed but it might help you for now.