@████████- you are amazing! That worked perfectly. Exactly what I was looking for! Thank you so much!
You are correct, I'm updating the checkbox labels via Javascript - I just inserted your code after I've done the label updates, and it sorts them perfectly.

If you're interested, here is the basics of how I'm re-doing the checkbox labels. My actual form is much more complex, but this is a simplified version that is easy to recreate:
First add a multi-line field on the form with CSS Class of "checkbox_values". Set its default value to "One|Two|Three|Four|DISABLED|DISABLED|Seven|Eight|DISABLED|Ten". This is what is being populated from my database (populated via Workflow) and this is what will serve the basis of the checkbox labels. The Javascript will label the checkboxes as those ten values that are separated by pipes ( | ) - if you don't want to use the numbers, you can use pretty much anything else. The code will also hide the three checkboxes that are called "DISABLED".
Second, add a checkbox field on the form with CSS Class of myCheckbox. Keep it set to a 1 column layout (that's all I've tested with at least). Give it ten choices called A, B, C, D, E, F, G, H, I, and J - copy the list of choices to the values, so they are identical.
Then here's the Javascript. This includes the code I'd already written to update the checkbox labels, and the code you provided to sort them alphabetically.
$(document).ready(function(){
//update all of the checkbox labels based on the value of the checkbox_values text box. Hide any checkboxes with a label of DISABLED.
var checkboxValues = $('.checkbox_values textarea').val().split('|');
var checkboxCounter = 0;
$('.myCheckbox .choice label').each(function() {
$(this).text(checkboxValues[checkboxCounter]);
if(checkboxValues[checkboxCounter] == 'DISABLED') {
$(this).parent().hide();
}
checkboxCounter++;
});
//sort the checkbox options alphabetically by their labels
$('.myCheckbox span.choice').parent().append($('.myCheckbox span.choice').detach().sort(function(a,b){
return $(a).find('.form-option-label').text().toUpperCase().localeCompare($(b).find('.form-option-label').text().toUpperCase());
}));
});
When you preview your form, you should see the checkbox with seven options, showing these labels and values:
Eight / H
Four / D
One / A
Seven / G
Ten / J
Three / C
Two / B
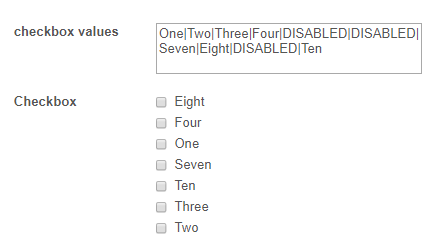
One thing to note however, for this to work with the archival version of the form (i.e. the version sent to Laserfiche) I do have to make a copy of the form with some small tweaks to the Javascript:
1. where it says "textarea" on line 4 gets replaced with ".cf-field" (alternately, if I'd used a single line field I would be using "input" instead of "textarea", but I would still change it to ".cf-field").
2. where it says "val()" on line 4 gets replaced with "text()"
By the way, this works the same for Checkboxes or Radio buttons. 