Can you clarify what you mean by output forms? Do you mean sequentially presenting the 5 follow-up forms to a user to review and click "Submit" on each one? Or do you mean save each of the 5 follow-up forms to the repository?
If you're trying to accomplish the first thing I mention, create a hidden single line "URL" field on your for and assign it the CSS class 'url'. Assign appropriate CSS classes to whatever fields you use in your lookup rules.
Assuming your process is kicked off by a Message Start Event, you can setup the "Thank You Message" tab to redirect the user to another URL, instead of the Thank You Message. The URL in this case should be the value of whatever is in the hidden URL field on your form--ie, use a token (see below).
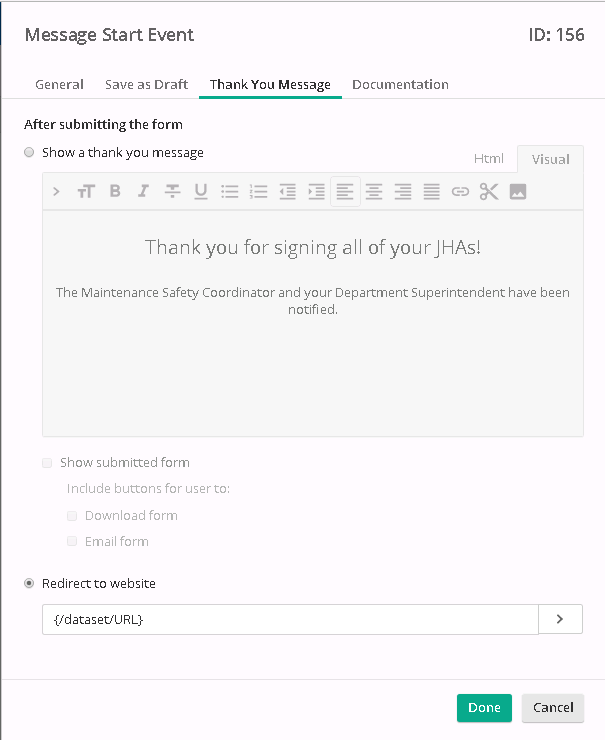
Then, you can modify the JavaScript snippet below to dynamically generate the URL of the "next" form in your set of output forms. This URL will populate your "URL" field. Once the final form in the set is submitted, the URL is set to some other website (your homepage, perhaps?) and a popup displays, thanking the user for submitting the forms. Uncomment the console.log lines if you like, for testing purposes.
$(document).ready(function() {
var url = $('.url input').val(),
yourField = $('.yourField2 input').val(),
yourField2 = $('.yourField2 select').val(),
urlAssignment = function() {
yourField = $('.yourField input').val();
// Remove 'x' anchors to delete table rows
$('.action a').text('');
// if statement checks for termination condition; your miles may vary
if (yourField2 == '') {
url = 'http://www.some.url.com/'; // After last form is submitted, user is redirected to this URL
$('.url input').val(url);
//console.log('variables initialized\nurl = ' + url + '\nyourField = ' + yourField + '\nyourField2 = ' + yourField2);
}
else {
url = 'http://111.111.111.11/Forms/YourFormNameGoesHere?Some_Variable=Some_Value&Your_Field='
+ encodeURIComponent(yourField) + '&Your_Field_2=' + encodeURIComponent(yourField);
$('.url input').val(url);
//console.log('variables initialized\nurl = ' + url + '\nyourField = ' + yourField + '\nyourField2 = ' + yourField2);
}
};
// Remove "Add" anchor below table
$('.hideAddRow').text('');
$('.yourField2 select').change(function() {
yourField2 = $(this).val();
//console.log('yourField3 changed to ' + yourField3);
});
$('.yourField input').on('change', function() {
setTimeout(urlAssignment, 250);
});
// Prevents display of popup message until last form is submitted with a signature (remove everything between the second single quote and the closing parenthesis if you don't need this)
$('.Submit').click(function() {
if (yourField2 != '' && $('.Sign_Sig').css('display') == 'none') {
alert('Thank you message goes here plus whatever field values (ie, ' + yourField + ' you like.');
}
});
setTimeout(urlAssignment, 250);
});
Sorry if all this is TMI--if it's not, I'm happy to answer any questions you might have.