On your coding chart table:
1. set the CSS class of the Table to: coding_chart_table
2. set the CSS class of the GL Code field to: codingGL
3. set the CSS class of the Amount field to: codingAmount
4. set the CSS class of the Status field to: codingStatus
5. make sure the Status field has an option called: Rejected
Add another table below your coding chart table:
1. set the CSS class of the Table to: rejected_items_table
2. add a single line field to the table with a label like Rejected Items
3. add some text to the "Text above field" setting on the Rejected Items field, I used "text goes here"
4. set the CSS class of the Rejected Items field to: rejectedField
It'll look something like this:
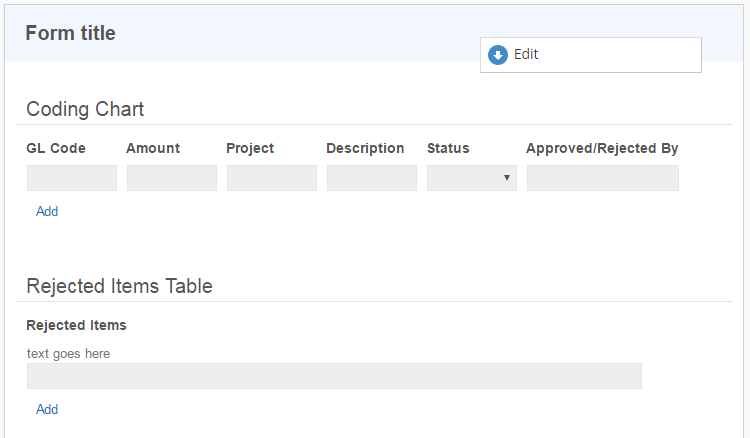
Add this as the CSS script for your form:
/*hide the header from the rejected items table (tweak appearance)
hide the add and delete buttons from the rejected items table (the system will
add and remove them to match with the coding chart table*/
.rejected_items_table .cf-section-header {display : none;}
.rejected_items_table .cf-table-add-row {display : none !important;}
.rejected_items_table .cf-table-delete {display : none !important;}
Add this as the Javascript for your form:
$(document).ready(function () {
//When the form loads, or changes are made to the coding table,
//run the function to update the rejected table values.
updateRejectedFields()
$('.coding_chart_table').on('change', function () {
updateRejectedFields()
}); //end of $('.coding_chart_table').on('change', function () {
//when a row is added to the coding chart table, add a row to the
//rejected items table too
$('.coding_chart_table').on('click', '.cf-table-add-row', function () {
$('.rejected_items_table .cf-table-add-row').trigger('click');
updateRejectedFields()
}); //end of $('.coding_chart_table').on('click', '.cf-table-add-row', function () {
//when a row is deleted from the coding chart table, delete the
//matching row from the rejected items table too, archive this
//by get index of the row being deleted, prior to the deletion
//processing, and then deleting that same indexed row from
//the other table.
var deleteIndex = -5;
$('.coding_chart_table').on('focusin', '.cf-table-delete', function () {
deleteIndex = $(this).closest('tr').index();
}); //end of $('.coding_chart_table').on('focusin', '.cf-table-delete', function () {
$('.coding_chart_table').on('click', '.cf-table-delete', function () {
var tableRowIndex = deleteIndex + 1;
$('.rejected_items_table tbody tr:nth-child(' + tableRowIndex + ')').find('.cf-table-delete').trigger('click');
updateRejectedFields()
}); //end of $('.coding_chart_table').on('click', '.cf-table-delete', function () {
//function that runs whenever the coding chart table is updated
//to ensure the rejected items table is updated to match
function updateRejectedFields() {
$('.codingStatus select').each(function() {
var theRowIndex = $(this).closest('tr').index();
var tableRowIndex = theRowIndex + 1;
if ($(this).val() == 'Rejected') {
var glValue = $(this).closest('tr').find('.codingGL input').val();
var amountValue = $(this).closest('tr').find('.codingAmount input').val();
var newText = 'Rejected Line Item: GL Code: ' + glValue + ', Amount: ' + amountValue + '. Please provide a reason.';
$('.rejected_items_table tbody tr:nth-child(' + tableRowIndex + ')').show();
$('.rejected_items_table tbody tr:nth-child(' + tableRowIndex + ')').find('.rejectedField label').text(newText);
}
else {
$('.rejected_items_table tbody tr:nth-child(' + tableRowIndex + ')').hide();
} //end of if...else... statement
}); //end of $('.codingStatus select').each(function() {
} //end of function updateRejectedFields() {
}); //end of $(document).ready(function () {
The completed form should look something like this:
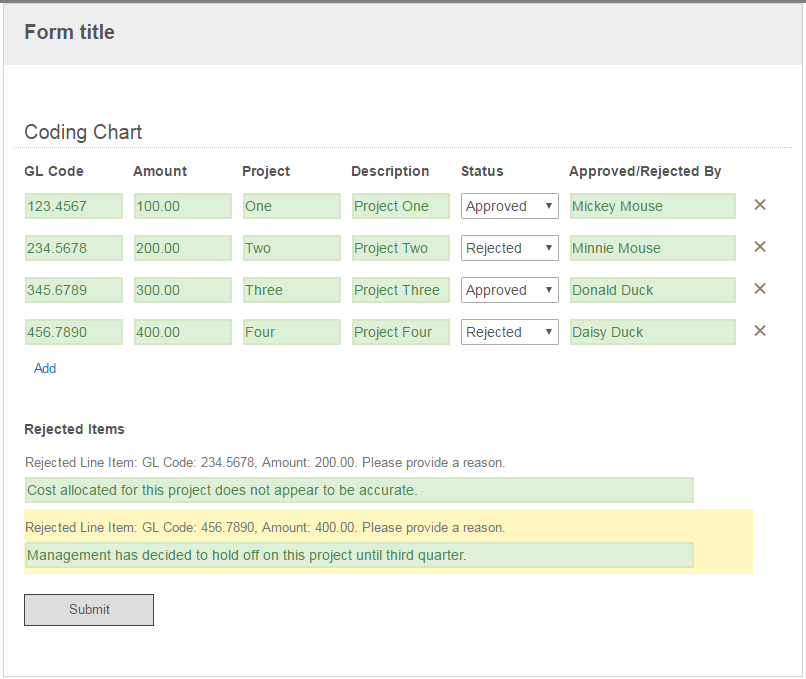