Here's how I've done it. This is using the Google Developers script for pie chart visualization (https://developers.google.com/chart/interactive/docs/gallery/piechart).
You'll need to store the loader.js file (https://www.gstatic.com/charts/loader.js ) in the js folder on your forms server (C:\Program Files\Laserfiche\Laserfiche Forms\Forms\js).
On my form, I added these elements:
1. Table - name: "Service Tasks" - variable: service_tasks_table - CSS Class: serviceTasksTable
1a. Table Column - type: Dropdown - name: Service Type - variable: service_type - CSS Class: serviceTask - Options: Window Cleaning, Table Cleaning, Floor Cleaning, Office Cleaning
1b. Table Column - type: Number - name: Hours Spent - variable: hours_spent - CSS Class: hoursSpent
2. Single Line Field - name: Window Cleaning - CSS Class: windowCleaningTotal hiddenField - fx: =SUMIF(service_tasks_table.service_type,"Window Cleaning",service_tasks_table.hours_spent)
3. Single Line Field - name: Table Cleaning - CSS Class: tableCleaningTotal hiddenField - fx: =SUMIF(service_tasks_table.service_type,"Table Cleaning",service_tasks_table.hours_spent)
4. Single Line Field - name: Floor Cleaning - CSS Class: floorCleaningTotal hiddenField - fx: =SUMIF(service_tasks_table.service_type,"Floor Cleaning",service_tasks_table.hours_spent)
5. Single Line Field - name: Office Cleaning - CSS Class: officeCleaningTotal hiddenField - fx: =SUMIF(service_tasks_table.service_type,"Office Cleaning",service_tasks_table.hours_spent)
6. Custom HTML - HTML: <div id="piechart" style="width: 100%; height: 400px;"></div>
It'll look like this:
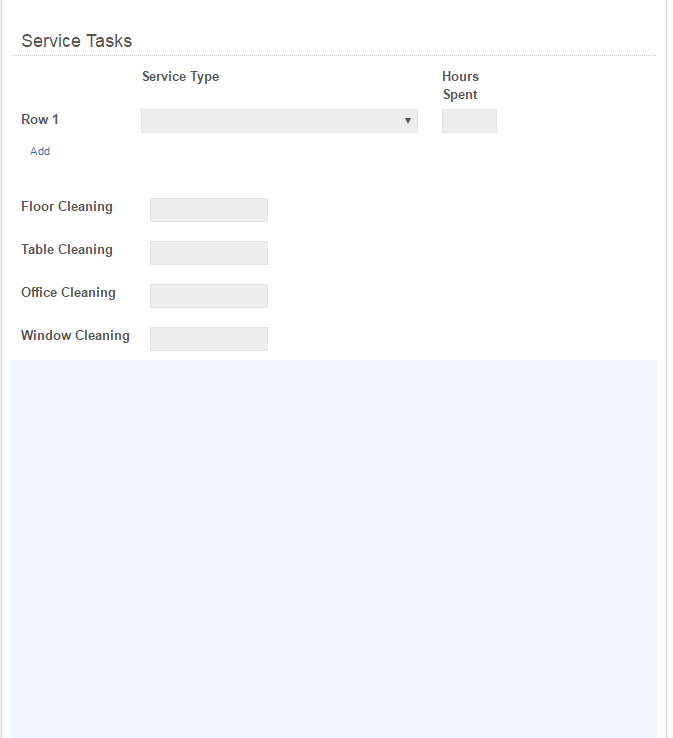
Then you'll add this CSS code (which will cause the four totals fields to be hidden):
/*hide any fields with the hiddenField Class*/
.hiddenField {display : none;}
Then you'll add this Javascript code (which will display and update the pie chart each time the Service Tasks table is updated):
$(document).ready(function() {
//load the Google Charts Visualization
$.getScript('http://FORMSERVER/forms/js/loader.js', function () {
});
//when anything in the Service Tasks Table changes, update the pie chart
$('.serviceTasksTable').on('change', function() {
//pie chart update code
google.charts.load('current', {'packages':['corechart']});
google.charts.setOnLoadCallback(drawChart);
function drawChart() {
//get the pie chart values from the hidden totals fields
var windowCleaning = parseInt($('.windowCleaningTotal input').val());
var floorCleaning = parseInt($('.floorCleaningTotal input').val());
var tableCleaning = parseInt($('.tableCleaningTotal input').val());
var officeCleaning = parseInt($('.officeCleaningTotal input').val());
//store the hidden totals fields into the array
var data = google.visualization.arrayToDataTable([
['Task','Hours'],
['Window Cleaning',windowCleaning],
['Table Cleaning',tableCleaning],
['Floor Cleaning',floorCleaning],
['Office Cleaning',officeCleaning]
]);
//set the chart title
var options = {
title: 'Service Tasks'
};
//update the pie chart
var chart = new google.visualization.PieChart(document.getElementById('piechart'));
chart.draw(data, options);
}
});
});
The final form should look like this:
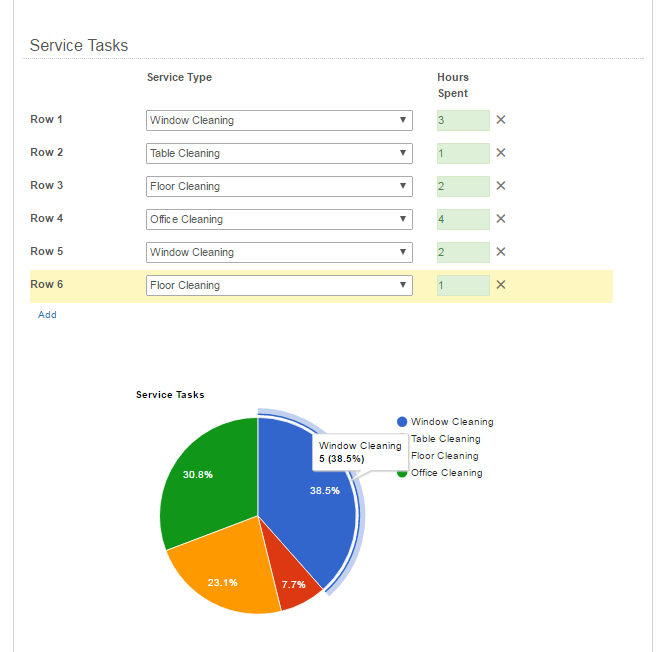